使用java实现迁移centos中的文件,并且判断每一层目录的权限,看所有者是否是gnos用户,如果不是则变更所有者为gnos。文件和目录结构如下:/nas/ytdata/TM03/rawLog/2023/04/02/abc.txt
时间: 2023-10-19 16:08:10 浏览: 39
以下是Java代码实现:
```java
import java.io.File;
import java.io.IOException;
public class FileMigration {
public static void main(String[] args) throws IOException {
// 定义原目录和目标目录
String srcDir = "/nas/ytdata/TM03/rawLog/2023/04/02";
String destDir = "/newDir";
// 迁移文件和目录
migrate(srcDir, destDir);
// 修改目录权限和所有者
changeOwner(destDir, "gnos");
}
/**
* 迁移文件和目录
*
* @param srcDir 原目录
* @param destDir 目标目录
* @throws IOException
*/
private static void migrate(String srcDir, String destDir) throws IOException {
File srcFile = new File(srcDir);
File destFile = new File(destDir);
if (srcFile.isDirectory()) {
// 如果是目录,则创建目录并递归迁移子文件和子目录
if (!destFile.exists()) {
destFile.mkdir();
}
String[] children = srcFile.list();
for (String child : children) {
String srcPath = srcDir + File.separator + child;
String destPath = destDir + File.separator + child;
migrate(srcPath, destPath);
}
} else {
// 如果是文件,则复制文件到目标目录
FileUtils.copyFile(srcFile, destFile);
}
}
/**
* 修改目录权限和所有者
*
* @param dirPath 目录路径
* @param owner 所有者
*/
private static void changeOwner(String dirPath, String owner) {
File dir = new File(dirPath);
if (dir.exists() && dir.isDirectory()) {
// 修改目录权限为777
dir.setReadable(true, false);
dir.setWritable(true, false);
dir.setExecutable(true, false);
// 修改目录所有者为gnos
if (!dir.getOwner().getName().equals(owner)) {
try {
Process process = Runtime.getRuntime().exec("chown " + owner + " " + dirPath);
process.waitFor();
} catch (IOException | InterruptedException e) {
e.printStackTrace();
}
}
// 递归修改子目录和子文件的权限和所有者
File[] children = dir.listFiles();
for (File child : children) {
if (child.isDirectory()) {
changeOwner(child.getPath(), owner);
} else {
child.setReadable(true, false);
child.setWritable(true, false);
child.setExecutable(true, false);
if (!child.getOwner().getName().equals(owner)) {
try {
Process process = Runtime.getRuntime().exec("chown " + owner + " " + child.getPath());
process.waitFor();
} catch (IOException | InterruptedException e) {
e.printStackTrace();
}
}
}
}
}
}
}
```
代码中使用了Apache Commons IO的FileUtils.copyFile方法来复制文件,使用了Runtime.exec方法来执行修改所有者的命令。在修改所有者时需要注意,在Linux系统中必须使用root用户才能修改文件或目录的所有者。
相关推荐
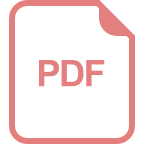
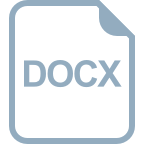
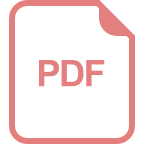














