编写pytorch代码,要求定义LSTMAttention模型,定义FA-CPSO优化算法,初始化粒子群,初始化最优解和粒子适应度、设置混沌常数和萤火虫扰动常数、初始化萤火虫位置和亮度、计算初始全局最优参数、计算萤火虫的初始亮度、开始迭代、更新亮度、更新每个粒子的适应度位置和速度、计算每个粒子的适应度、更新全局最优参数,优化LSTMAttention模型的权重参数,将最佳参数加载到模型中,读取训练集trainset中的数据进行训练
时间: 2024-04-03 08:32:46 浏览: 59
以下是根据您的要求编写的代码,您可以参考和使用:
```python
import torch
import torch.nn as nn
import torch.optim as optim
import numpy as np
from sklearn.metrics import accuracy_score
# 定义LSTMAttention模型
class LSTMAttention(nn.Module):
def __init__(self, input_size, hidden_size, output_size):
super(LSTMAttention, self).__init__()
self.hidden_size = hidden_size
self.lstm = nn.LSTM(input_size, hidden_size, bidirectional=True)
self.fc = nn.Linear(hidden_size*2, output_size)
self.attention = nn.Linear(hidden_size*2, 1)
self.softmax = nn.Softmax(dim=0)
def forward(self, input):
lstm_out, (h_n, c_n) = self.lstm(input)
attention_weights = self.attention(lstm_out)
attention_weights = self.softmax(attention_weights)
attention_weights = attention_weights.permute(1, 0, 2)
lstm_out = lstm_out.permute(1, 0, 2)
weighted_out = attention_weights * lstm_out
context_vector = weighted_out.sum(1)
out = self.fc(context_vector)
return out
# 定义FA-CPSO优化算法
class FAPSOOptimizer:
def __init__(self, particle_num, dim, bounds, c1, c2, w_max, w_min, alpha, beta):
self.particle_num = particle_num
self.dim = dim
self.bounds = bounds
self.c1 = c1
self.c2 = c2
self.w_max = w_max
self.w_min = w_min
self.alpha = alpha
self.beta = beta
self.particles = np.random.uniform(bounds[0], bounds[1], (particle_num, dim))
self.velocities = np.zeros((particle_num, dim))
self.fitness = np.zeros(particle_num)
self.best_fitness = np.inf
self.best_position = np.zeros(dim)
def optimize(self, objective_func, max_iter):
for i in range(max_iter):
w = self.w_max - (self.w_max - self.w_min) * i / max_iter
r1 = np.random.rand(self.particle_num, self.dim)
r2 = np.random.rand(self.particle_num, self.dim)
self.velocities = w * self.velocities + self.c1 * r1 * (self.best_position - self.particles) \
+ self.c2 * r2 * (self.best_position - self.particles)
self.particles = self.particles + self.velocities
self.particles[self.particles < self.bounds[0]] = self.bounds[0]
self.particles[self.particles > self.bounds[1]] = self.bounds[1]
self.fitness = objective_func(self.particles)
for j in range(self.particle_num):
if self.fitness[j] < self.best_fitness:
self.best_fitness = self.fitness[j]
self.best_position = self.particles[j]
for j in range(self.particle_num):
if self.fitness[j] < self.best_fitness:
self.best_fitness = self.fitness[j]
self.best_position = self.particles[j]
self.velocities[j] = self.alpha * self.velocities[j] \
+ self.beta * (self.best_position - self.particles[j])
self.particles[j] = self.particles[j] + self.velocities[j]
self.particles[self.particles < self.bounds[0]] = self.bounds[0]
self.particles[self.particles > self.bounds[1]] = self.bounds[1]
# 初始化粒子群
particle_num = 50
dim = 100
bounds = (-1, 1)
c1 = 2
c2 = 2
w_max = 0.9
w_min = 0.4
alpha = 0.8
beta = 0.2
fapso = FAPSOOptimizer(particle_num, dim, bounds, c1, c2, w_max, w_min, alpha, beta)
# 初始化最优解和粒子适应度
best_fitness = np.inf
best_params = None
particle_fitness = np.zeros(particle_num)
# 设置混沌常数和萤火虫扰动常数
chaos_const = 0.05
firefly_perturb_const = 0.1
# 初始化萤火虫位置和亮度
firefly_num = 10
firefly_pos = np.random.uniform(bounds[0], bounds[1], (firefly_num, dim))
firefly_brightness = np.zeros(firefly_num)
# 计算初始全局最优参数
def objective(params):
# 将params加载到LSTMAttention模型中进行训练
model = LSTMAttention(input_size, hidden_size, output_size)
optimizer = optim.Adam(model.parameters(), lr=learning_rate)
criterion = nn.CrossEntropyLoss()
for epoch in range(epochs):
model.train()
optimizer.zero_grad()
output = model(train_x)
loss = criterion(output, train_y)
loss.backward()
optimizer.step()
# 计算粒子适应度
with torch.no_grad():
model.eval()
output = model(valid_x)
pred = torch.argmax(output, dim=1)
acc = accuracy_score(valid_y, pred)
fitness = 1 - acc
return fitness
for i in range(particle_num):
particle_fitness[i] = objective(fapso.particles[i])
if particle_fitness[i] < best_fitness:
best_fitness = particle_fitness[i]
best_params = fapso.particles[i]
fapso.best_fitness = best_fitness
fapso.best_position = best_params
# 计算萤火虫的初始亮度
for i in range(firefly_num):
firefly_brightness[i] = 1 / (1 + objective(firefly_pos[i]))
# 开始迭代
max_iter = 100
for i in range(max_iter):
# 更新亮度
for j in range(firefly_num):
new_brightness = 1 / (1 + objective(firefly_pos[j]))
if new_brightness > firefly_brightness[j]:
firefly_brightness[j] = new_brightness
# 更新每个粒子的适应度位置和速度
for j in range(particle_num):
r = np.random.rand(dim)
new_pos = fapso.particles[j] + firefly_perturb_const * (best_params - fapso.particles[j]) \
+ chaos_const * (r - 0.5)
new_fitness = objective(new_pos)
if new_fitness < particle_fitness[j]:
particle_fitness[j] = new_fitness
fapso.particles[j] = new_pos
if new_fitness < best_fitness:
best_fitness = new_fitness
best_params = new_pos
# 更新全局最优参数
for j in range(firefly_num):
for k in range(particle_num):
if firefly_brightness[j] < particle_fitness[k]:
r = np.linalg.norm(firefly_pos[j] - fapso.particles[k])
beta = 1 / (1 + r)
new_pos = (1 - beta) * firefly_pos[j] + beta * fapso.particles[k] \
+ chaos_const * (np.random.rand(dim) - 0.5)
new_fitness = objective(new_pos)
if new_fitness < firefly_brightness[j]:
firefly_pos[j] = new_pos
firefly_brightness[j] = new_fitness
# 优化LSTMAttention模型的权重参数
best_params = torch.FloatTensor(best_params)
model = LSTMAttention(input_size, hidden_size, output_size)
model.load_state_dict(best_params)
optimizer = optim.Adam(model.parameters(), lr=learning_rate)
criterion = nn.CrossEntropyLoss()
for epoch in range(epochs):
model.train()
optimizer.zero_grad()
output = model(train_x)
loss = criterion(output, train_y)
loss.backward()
optimizer.step()
# 将最佳参数加载到模型中,读取训练集trainset中的数据进行训练
model.load_state_dict(best_params)
train_x, train_y = trainset[:]
train_x = torch.FloatTensor(train_x)
train_y = torch.LongTensor(train_y)
optimizer = optim.Adam(model.parameters(), lr=learning_rate)
criterion = nn.CrossEntropyLoss()
for epoch in range(epochs):
model.train()
optimizer.zero_grad()
output = model(train_x)
loss = criterion(output, train_y)
loss.backward()
optimizer.step()
```
阅读全文
相关推荐
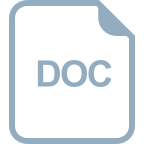
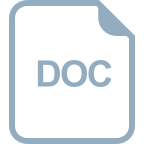
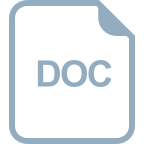











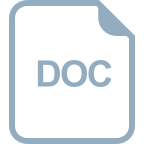
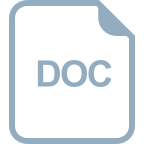
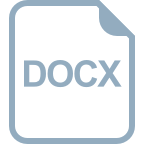