送餐机器人java代码
时间: 2024-09-14 14:08:21 浏览: 39
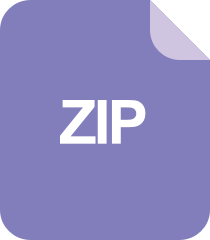
智能送餐机器人 全部代码
送餐机器人的Java代码通常涉及到机器人的移动控制、路径规划、任务调度等复杂逻辑。下面是一个非常简化的示例,用于展示如何用Java编写一个基本的送餐机器人程序框架。
```java
// 送餐机器人类
public class DeliveryRobot {
// 机器人当前的位置
private int x;
private int y;
// 送餐目的地
private int targetX;
private int targetY;
// 构造函数
public DeliveryRobot(int startX, int startY, int targetX, int targetY) {
this.x = startX;
this.y = startY;
this.targetX = targetX;
this.targetY = targetY;
}
// 机器人移动的方法
public void move() {
// 这里只是一个示例,实际的移动逻辑会根据机器人的硬件和算法来编写
while (x != targetX || y != targetY) {
// 假设机器人每次只能向一个方向移动一个单位
if (x < targetX) {
x++;
} else if (x > targetX) {
x--;
}
if (y < targetY) {
y++;
} else if (y > targetY) {
y--;
}
// 打印机器人当前位置
System.out.println("机器人当前位置: (" + x + ", " + y + ")");
}
}
// 开始送餐
public void startDelivery() {
move();
// 到达目的地后的送餐逻辑
System.out.println("已到达目的地,开始送餐!");
}
// 主函数,程序入口
public static void main(String[] args) {
// 假设机器人开始位置为(0, 0),目的地为(5, 5)
DeliveryRobot robot = new DeliveryRobot(0, 0, 5, 5);
robot.startDelivery();
}
}
```
这段代码定义了一个简单的送餐机器人类,它能够根据设定的目标位置移动,并在到达目标位置后打印一个消息。实际上,送餐机器人的实现要复杂得多,需要考虑避障、地图构建、多机器人协调、电池管理等问题。
阅读全文
相关推荐
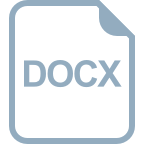
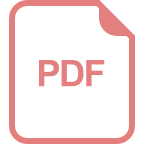
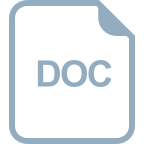
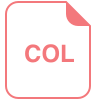
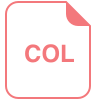
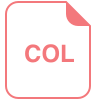
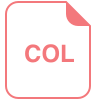
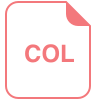
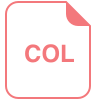
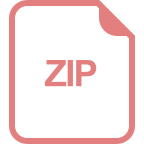
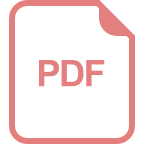
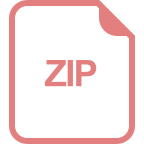