设计一个程序为参加歌手大赛的选手计算最终得分。评委给出的分数是 0~10 分。选手最后得分:去掉一个最高分,去掉一个最低分,计算其余评委打分的平均值。用python语言
时间: 2024-06-13 20:06:50 浏览: 108
以下是Python程序,用于计算参加歌手大赛的选手最终得分:
```python
scores = []
num_judges = int(input("请输入评委人数:"))
for i in range(num_judges):
score = float(input("请输入第{}位评委的分数:".format(i+1)))
scores.append(score)
scores.sort()
scores = scores[1:-1] # 去掉一个最高分和一个最低分
average_score = sum(scores) / len(scores)
below_average = sum(1 for score in scores if score < average_score)
print("选手最终得分为:{:.2f}".format(average_score))
print("有{}位评委给了他低于平均分的成绩。".format(below_average))
```
相关问题
设计一个程序为参加歌手大赛的选手计算最终得分。评委给出的分数是 0~10 分。选手最后得分:去掉一个最高分,去掉一个最低分,计算其余评委打分的平
以下是Python程序,用于计算参加歌手大赛的选手最终得分:
```python
scores = []
num_judges = int(input("请输入评委人数:"))
for i in range(num_judges):
score = float(input("请输入第{}位评委的分数:".format(i+1)))
scores.append(score)
scores.sort()
scores = scores[1:-1]
average_score = sum(scores) / len(scores)
print("选手最终得分为:{:.2f}".format(average_score))
```
设计一个程序为参加歌手大赛的选手计算最终得分。评委给出的分数是0~10 分。选手最后得分:去掉一个最高分去掉一个最低分,计算其余评委打分的平均值。
设计这样一个程序可以分为以下几个步骤:
1. **初始化数据结构**:首先,你需要创建一个列表来存储每个评委对每个选手的评分。例如,使用字典来表示每个选手和其对应的评分列表。
```python
scores = {}
```
2. **收集评委评分**:每次收到评委的评分时,更新选手的字典,键是选手名,值是包含所有评分的列表。
```python
def add_score(competitor, judge_score):
if competitor not in scores:
scores[competitor] = []
scores[competitor].append(judge_score)
```
3. **计算最终得分**:对于每个选手,找到最高分和最低分,然后计算剩余评分的平均值。
```python
def calculate_final_score(competitor):
scores_list = scores.get(competitor, [])
if len(scores_list) < 2: # 至少需要两个评分
return None
highest_score = max(scores_list)
lowest_score = min(scores_list)
filtered_scores = [score for score in scores_list if score != highest_score and score != lowest_score]
average_score = sum(filtered_scores) / (len(filtered_scores) if filtered_scores else 1)
return average_score
```
4. **主程序循环**:如果有多位选手参赛,可以遍历每个选手并调用 `calculate_final_score` 函数。
5. **展示结果**:最后,你可以打印出每位选手的名称及其最终得分。
```python
for competitor, scores in scores.items():
final_score = calculate_final_score(competitor)
if final_score is not None:
print(f"{competitor} 的最终得分是:{final_score}")
```
阅读全文
相关推荐
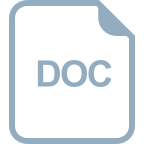











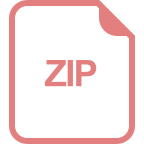