请你重写,代码不够详细
时间: 2024-10-25 19:15:48 浏览: 23
当然,我很抱歉之前的描述不够详尽。让我们逐步细化每个部分的代码实现。
**1. 定义Book类**
```python
class Book:
def __init__(self, id: int, title: str, author: str, publication_year: int):
self._id = id
self._title = title
self._author = author
self._publication_year = publication_year
self.is_borrowed = False
@property
def id(self) -> int:
return self._id
@id.setter
def id(self, value: int) -> None:
self._id = value
# 添加getter和setter方法,例如:
@property
def title(self) -> str:
return self._title
@title.setter
def title(self, value: str) -> None:
self._title = value
# 借书和还书方法
def borrow_book(self):
if not self.is_borrowed:
self.is_borrowed = True
print("Book successfully borrowed.")
else:
print("The book is already borrowed.")
def return_book(self):
if self.is_borrowed:
self.is_borrowed = False
print("Book returned.")
else:
print("Book was not borrowed.")
def display_info(self):
print(f"ID: {self.id}, Title: {self.title}, Author: {self.author}, Publication Year: {self.publication_year}, Borrowed: {'Yes' if self.is_borrowed else 'No'}")
```
**2. 定义Library类**
```python
class Library:
def __init__(self):
self.books = []
# 添加书籍方法
def add_book(self, book: Book) -> None:
self.books.append(book)
# 删除书籍方法
def remove_book(self, book_id: int) -> bool:
for idx, book in enumerate(self.books):
if book.id == book_id:
del self.books[idx]
return True
return False
# 更新书籍方法
def update_book(self, old_id: int, new_book: Book) -> bool:
updated = False
for idx, book in enumerate(self.books):
if book.id == old_id:
self.books[idx] = new_book
updated = True
break
return updated
# 保存和加载到文件的方法
def save_to_file(self, filename: str) -> None:
with open(filename, 'w', newline='', encoding='utf-8') as f:
json.dump([book.__dict__ for book in self.books], f)
def load_from_file(self, filename: str) -> None:
try:
with open(filename, 'r', encoding='utf-8') as f:
self.books = [Book(**data) for data in json.load(f)]
except Exception as e:
print(f"Error loading from file: {e}")
# CLI接口
def cli_interact(self):
while True:
# 显示菜单并获取用户输入
# ...此处实现具体命令处理逻辑...
# 示例:
command = input("Enter your command (add, remove, search, borrow, return, quit): ")
if command == "quit":
break
elif command == "add":
# 添加图书
elif command == "remove":
# 删除图书
elif command == "borrow":
# 借书
elif command == "return":
# 还书
# ...处理其他命令...
```
**4. 异常处理**
```python
# 在处理文件操作的地方添加异常处理,如:
try:
self.save_to_file('library.json')
except (IOError, FileNotFoundError) as e:
print(f"An error occurred during file operation: {str(e)}")
```
**5. 用户交互**
```python
def cli_interact(self):
while True:
# 用户输入验证
command, *args = input("Enter your command followed by book ID or details (example: add 1 MyBook John Doe 2020): ").split()
if command.lower() == "exit":
break
# 分别处理各条命令
if command.lower() == "add":
# 解析输入并添加图书
elif command.lower() == "remove":
# 解析输入并删除图书
# ...处理其他命令...
```
现在代码已经更详细了,你可以按照这个结构继续完善每个命令的处理以及异常处理。如果还有其他关于如何实现特定功能的问题,请随时提问。
阅读全文
相关推荐
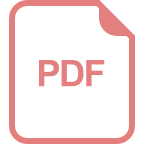
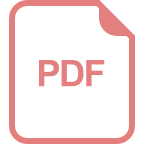
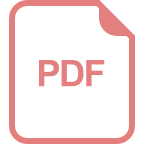
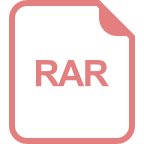
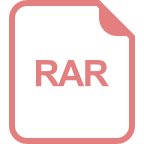
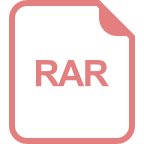
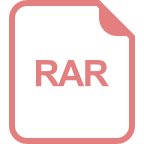
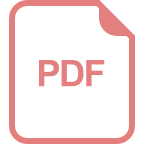
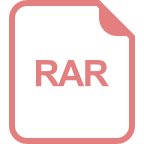
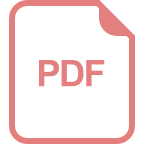
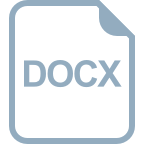
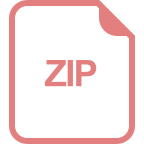
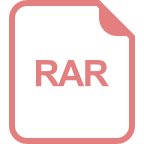
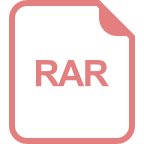
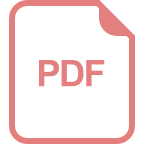
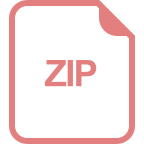
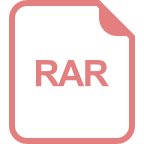
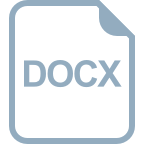