出现报错:AttributeError: 'DataFrame' object has no attribute 'reshape'
时间: 2023-08-02 17:08:35 浏览: 122
这个错误通常发生在尝试在 `DataFrame` 对象上调用 `reshape` 函数时。`DataFrame` 是 pandas 库中的一个数据结构,它不直接支持 `reshape` 操作。要解决这个问题,你需要将 `DataFrame` 转换为 NumPy 数组,然后再进行 `reshape` 操作。
以下是一个示例代码,展示如何将 `DataFrame` 对象转换为 NumPy 数组并进行 `reshape` 操作:
```python
import numpy as np
import pandas as pd
# 假设你的数据存储在名为 df 的 DataFrame 对象中
# df = pd.DataFrame(...) # 加载数据集到 DataFrame
# 将 DataFrame 转换为 NumPy 数组
X = df.to_numpy()
# 进行 reshape 操作
X_reshaped = X.reshape(num_samples, num_features)
# 继续进行后续操作,例如模型训练等
```
在上述代码中,我们使用 `to_numpy()` 函数将 `DataFrame` 对象 `df` 转换为 NumPy 数组 `X`。然后,我们可以对 `X` 进行 `reshape` 操作,将其转换为期望的形状。
请确保已经导入了 `numpy` 和 `pandas` 库,并根据你的实际需求修改和应用上述代码。希望这个解决方案能够帮助你解决报错问题。
阅读全文
相关推荐















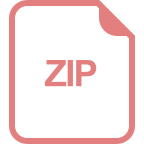