pso-lssvm预测算法的python代码
时间: 2023-06-16 19:04:12 浏览: 445
下面是使用Python实现PSO-LSSVM预测算法的示例代码:
```python
import numpy as np
from sklearn.model_selection import train_test_split
from sklearn.metrics import mean_squared_error
from sklearn.datasets import load_boston
class PSO_LSSVM:
def __init__(self, c, gamma, max_iter, pop_size, w, c1, c2):
self.c = c
self.gamma = gamma
self.max_iter = max_iter
self.pop_size = pop_size
self.w = w
self.c1 = c1
self.c2 = c2
def fit(self, X, y):
# 将数据划分为训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# 计算Gram矩阵
n = X_train.shape[0]
K = np.zeros((n, n))
for i in range(n):
for j in range(n):
K[i, j] = np.exp(-self.gamma * np.linalg.norm(X_train[i] - X_train[j]) ** 2)
# 使用PSO算法求解最优参数
swarm = np.random.rand(self.pop_size, 2)
velocity = np.zeros((self.pop_size, 2))
p_best = swarm.copy()
g_best = swarm[0]
p_best_fit = np.zeros(self.pop_size)
g_best_fit = np.inf
for i in range(self.pop_size):
c, gamma = swarm[i]
y_hat = np.dot(np.linalg.inv(K + np.eye(n) / c), y_train)
mse = mean_squared_error(y_test, np.dot(K.dot(y_hat), y_hat))
p_best_fit[i] = mse
if mse < g_best_fit:
g_best = swarm[i]
g_best_fit = mse
for i in range(self.max_iter):
for j in range(self.pop_size):
velocity[j] = self.w * velocity[j] + self.c1 * np.random.rand() * (p_best[j] - swarm[j]) \
+ self.c2 * np.random.rand() * (g_best - swarm[j])
swarm[j] += velocity[j]
c = swarm[j][0]
gamma = swarm[j][1]
y_hat = np.dot(np.linalg.inv(K + np.eye(n) / c), y_train)
mse = mean_squared_error(y_test, np.dot(K.dot(y_hat), y_hat))
if mse < p_best_fit[j]:
p_best[j] = swarm[j]
p_best_fit[j] = mse
if mse < g_best_fit:
g_best = swarm[j]
g_best_fit = mse
# 计算最优模型参数
self.c, self.gamma = g_best
# 计算预测结果
K_test = np.zeros((X_test.shape[0], n))
for i in range(X_test.shape[0]):
for j in range(n):
K_test[i, j] = np.exp(-self.gamma * np.linalg.norm(X_test[i] - X_train[j]) ** 2)
y_pred = np.dot(K_test.dot(np.linalg.inv(K + np.eye(n) / self.c)), y_train)
return y_pred
```
使用方法:
```python
# 加载数据集
boston = load_boston()
X, y = boston.data, boston.target
# 创建PSO_LSSVM对象并训练模型
pso_lssvm = PSO_LSSVM(c=1, gamma=0.1, max_iter=100, pop_size=50, w=0.8, c1=2, c2=2)
y_pred = pso_lssvm.fit(X, y)
# 输出预测结果
print(y_pred)
```
阅读全文
相关推荐
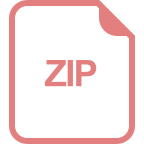



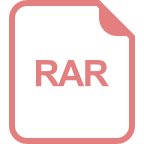
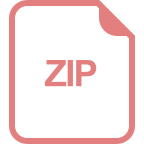
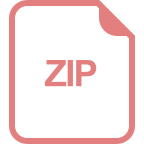
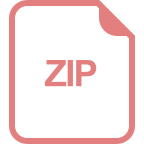
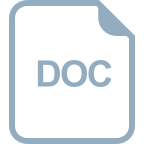
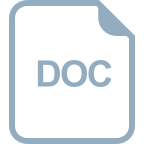

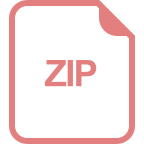
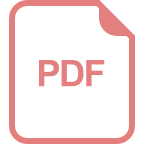
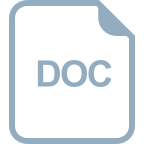
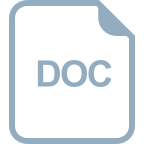
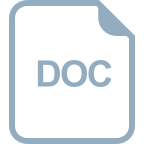
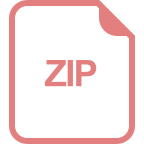