Netty服务端发送消息到客户端java源码
时间: 2023-10-03 18:02:20 浏览: 169
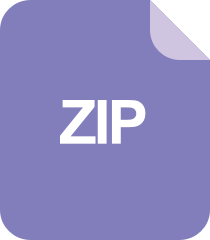
Netty服务端与客户端依靠Java序列化传输数据
以下是一个简单的 Netty 服务端发送消息到客户端的 Java 代码示例:
```java
import io.netty.bootstrap.ServerBootstrap;
import io.netty.channel.ChannelFuture;
import io.netty.channel.ChannelHandlerContext;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.ChannelPipeline;
import io.netty.channel.SimpleChannelInboundHandler;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.SocketChannel;
import io.netty.channel.socket.nio.NioServerSocketChannel;
import io.netty.handler.codec.string.StringDecoder;
import io.netty.handler.codec.string.StringEncoder;
public class NettyServer {
private int port;
public NettyServer(int port) {
this.port = port;
}
public void run() throws Exception {
NioEventLoopGroup bossGroup = new NioEventLoopGroup();
NioEventLoopGroup workerGroup = new NioEventLoopGroup();
try {
ServerBootstrap bootstrap = new ServerBootstrap();
bootstrap.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
ChannelPipeline pipeline = ch.pipeline();
pipeline.addLast(new StringDecoder());
pipeline.addLast(new StringEncoder());
pipeline.addLast(new NettyServerHandler());
}
});
ChannelFuture future = bootstrap.bind(port).sync();
future.channel().closeFuture().sync();
} finally {
bossGroup.shutdownGracefully();
workerGroup.shutdownGracefully();
}
}
public static void main(String[] args) throws Exception {
new NettyServer(8080).run();
}
private class NettyServerHandler extends SimpleChannelInboundHandler<String> {
@Override
protected void channelRead0(ChannelHandlerContext ctx, String msg) throws Exception {
// 接收到客户端的消息
System.out.println("接收到客户端的消息:" + msg);
// 向客户端发送消息
ctx.writeAndFlush("服务端已接收到消息:" + msg);
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) throws Exception {
cause.printStackTrace();
ctx.close();
}
}
}
```
在上述代码中,我们创建了一个 Netty 服务端,并且在 `NettyServerHandler` 类中实现了处理客户端消息的方法 `channelRead0`。在该方法中,我们打印了客户端发送的消息,并且使用 `ctx.writeAndFlush` 向客户端发送了一个回应消息。需要注意的是,在 Netty 中,所有的网络操作都是异步的,因此我们需要使用 `ChannelFuture` 来等待异步操作完成。
阅读全文
相关推荐
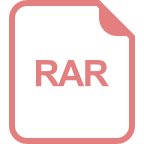
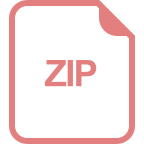
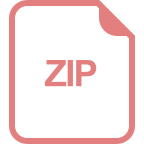
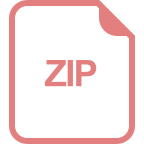
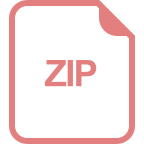
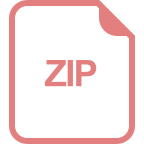
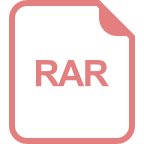
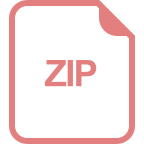
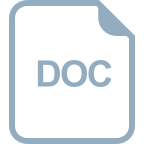
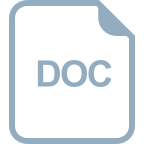
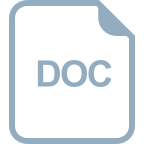
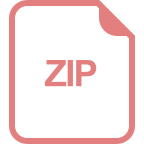
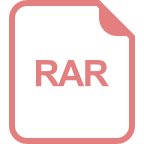
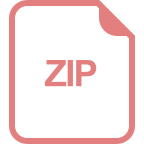
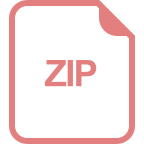
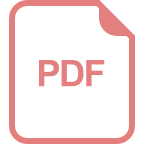
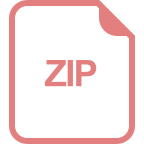