#include <stdio.h> #include <ctype.h> void main() { int cout_num,count_space,count_letter=0; int i,j; int line[]; for(i=0;i<3;i++) { pfintf("请输入第%d行文字",i+1); fgets(line,100); for(j=0;j<sizeof(line);j++) { if(isdigit(line[j])) { cout_num++; } else if(isalpa(line[j])) { count_letter++; } else if(line[j]==' ') { count_space++; } } } printf("数字%d,字母%d,空格%d",cout_num,count_letter,count_space); }有问题吗
时间: 2024-01-14 14:04:52 浏览: 61
有几个问题:
1. `main` 函数应该有返回值类型,应该改为 `int main()`。
2. `pfintf` 应该是 `printf` 的拼写错误,应该改为 `printf`。
3. 在 `fgets` 函数中,应该将 `line` 数组的大小指定为 100,即 `fgets(line,100,stdin)`。
4. 在 `sizeof` 函数中,应该使用数组名 `line`,而不是数组的地址 `&line`。
5. 在循环中需要将计数变量初始化为 0,即 `int cout_num=0,count_space=0,count_letter=0;`。
6. 在循环中需要清空 `line` 数组中的内容,可以使用 `memset(line,0,sizeof(line));`。
7. 在输出结果时,应该换行输出,即 `printf("数字%d,字母%d,空格%d\n",cout_num,count_letter,count_space);`。
综上所述,修正后的代码如下:
```
#include <stdio.h>
#include <ctype.h>
#include <string.h>
int main() {
int cout_num=0, count_space=0, count_letter=0;
int i, j;
char line[100];
for (i=0; i<3; i++) {
printf("请输入第%d行文字:", i+1);
fgets(line, 100, stdin);
memset(line, 0, sizeof(line));
for (j=0; j<strlen(line); j++) {
if (isdigit(line[j])) {
cout_num++;
} else if (isalpha(line[j])) {
count_letter++;
} else if (line[j]==' ') {
count_space++;
}
}
}
printf("数字%d,字母%d,空格%d\n", cout_num, count_letter, count_space);
return 0;
}
```
阅读全文
相关推荐
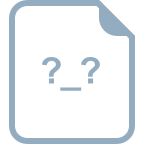
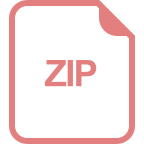
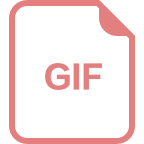
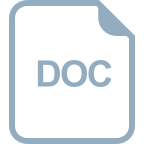
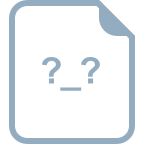
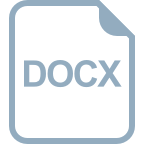
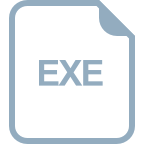
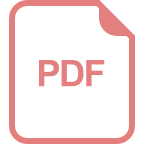
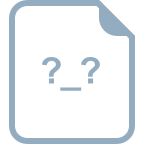
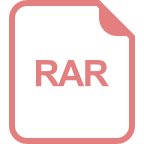
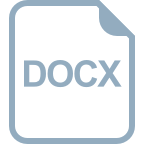
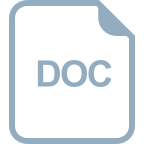
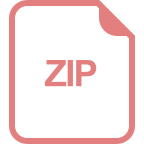
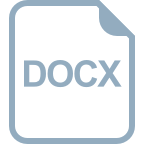
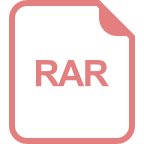
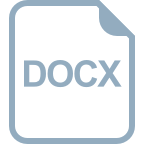