c++不用swap函数和sort函数,写代码将输入的三个整数从小到大输出
时间: 2023-03-21 20:04:00 浏览: 76
可以使用冒泡排序的方法将三个整数从小到大排序,代码如下:
```c++
#include <iostream>
using namespace std;
int main()
{
int a, b, c;
cin >> a >> b >> c;
// 冒泡排序
if (a > b) {
int temp = a;
a = b;
b = temp;
}
if (a > c) {
int temp = a;
a = c;
c = temp;
}
if (b > c) {
int temp = b;
b = c;
c = temp;
}
// 输出结果
cout << a << " " << b << " " << c << endl;
return 0;
}
```
以上代码中,首先通过 `cin` 语句输入三个整数 `a`、`b` 和 `c`,然后通过三次判断和交换的方式,将它们从小到大排序,最后通过 `cout` 语句输出排序后的结果。
相关问题
c++不用swap函数和sort函数以及冒泡,只用ifelse语句写代码将输入的三个整数从小到大输出
可以使用以下的代码实现将输入的三个整数从小到大输出:
```
#include <stdio.h>
int main() {
int a, b, c;
scanf("%d %d %d", &a, &b, &c);
if (a > b) {
int temp = a;
a = b;
b = temp;
}
if (a > c) {
int temp = a;
a = c;
c = temp;
}
if (b > c) {
int temp = b;
b = c;
c = temp;
}
printf("%d %d %d", a, b, c);
return 0;
}
```
代码思路:先输入三个整数,然后使用 if-else 语句进行判断,将三个数从小到大排序。
代码在主函数中输入10个整数,调用函数sort将它们按从小到大的顺序排序,排序结果在主函数中输出。
好的,以下是实现代码:
```c++
#include <iostream>
#include <algorithm>
using namespace std;
void sort(int arr[], int len) {
for(int i=0; i<len; i++) {
for(int j=i+1; j<len; j++) {
if(arr[i] > arr[j]) {
swap(arr[i], arr[j]);
}
}
}
}
int main() {
int arr[10];
cout << "请输入10个整数:" << endl;
for(int i=0; i<10; i++) {
cin >> arr[i];
}
sort(arr, 10);
cout << "排序后的结果为:" << endl;
for(int i=0; i<10; i++) {
cout << arr[i] << " ";
}
cout << endl;
return 0;
}
```
以上代码中,我们首先在主函数中输入了10个整数,然后调用了`sort`函数对它们进行排序,最后在主函数中输出排序后的结果。`sort`函数使用了冒泡排序算法,将数组中的元素按从小到大的顺序排序。
相关推荐
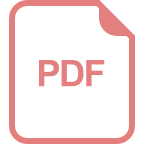












