condition_variable 怎么使用?
时间: 2023-09-01 12:09:13 浏览: 136
condition_variable 是 C++11 中的一个线程同步工具,用于实现线程间的条件变量通信。它通常与 std::mutex(互斥量)和 std::unique_lock(独占锁)一起使用。
使用 condition_variable 需要以下步骤:
1. 定义一个 std::condition_variable 对象,用于线程间的条件变量通信。
2. 定义一个 std::mutex 对象,用于保护共享资源。
3. 在一个线程中,通过 std::unique_lock<std::mutex> 对象锁住互斥量,然后等待条件满足时被唤醒。
4. 在另一个线程中,通过 std::unique_lock<std::mutex> 对象锁住互斥量,然后满足条件,并通过 condition_variable 的 notify_one() 或 notify_all() 方法唤醒等待的线程。
下面是一个简单的示例代码:
```cpp
#include <iostream>
#include <thread>
#include <condition_variable>
std::condition_variable cv;
std::mutex mtx;
bool ready = false;
void worker_thread()
{
std::cout << "Worker thread is waiting..." << std::endl;
std::unique_lock<std::mutex> lock(mtx);
cv.wait(lock, []{ return ready; }); // 等待条件满足时被唤醒
std::cout << "Worker thread is awake!" << std::endl;
}
int main()
{
std::thread worker(worker_thread);
// 做一些其他工作...
std::this_thread::sleep_for(std::chrono::seconds(2));
{
std::lock_guard<std::mutex> lock(mtx);
ready = true;
}
cv.notify_one(); // 唤醒等待的线程
worker.join();
return 0;
}
```
在上面的示例中,worker_thread() 函数是一个工作线程,它首先锁住互斥量,然后通过 cv.wait() 等待条件满足时被唤醒。在主线程中,先进行一些其他工作,然后通过锁住互斥量并设置 ready 变量为 true,最后通过 cv.notify_one() 唤醒等待的线程。
需要注意的是,condition_variable 的 wait() 方法需要接收一个可调用对象作为条件判断函数,用于判断条件是否满足。当条件返回 false 时,wait() 方法将线程阻塞并释放互斥量,直到条件为 true 时被唤醒并重新获取互斥量。
阅读全文
相关推荐
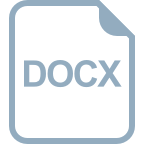
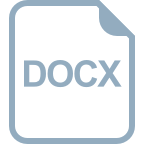
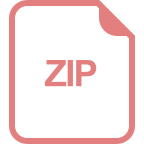


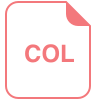










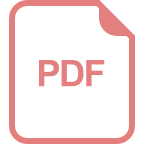
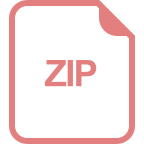