用c语言格式数组去重。要求编写去重的函数。
时间: 2024-09-13 22:15:56 浏览: 77
在C语言中,由于数组的元素一旦初始化就不能动态改变大小,所以我们通常使用其他数据结构如链表或者哈希表来实现数组的去重。这里我们以使用链表为例,创建一个简单的函数来去除一维数组中的重复元素:
```c
#include <stdio.h>
#include <stdlib.h> // 需要包含malloc和free函数
// 定义链表节点结构体
typedef struct Node {
int data;
struct Node* next;
} Node;
// 创建新节点
Node* createNode(int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (newNode != NULL) {
newNode->data = value;
newNode->next = NULL;
}
return newNode;
}
// 去除数组中的重复元素
void removeDuplicates(int arr[], int n) {
Node* head = NULL;
for (int i = 0; i < n; i++) {
Node* current = head;
while (current != NULL && current->data != arr[i]) {
current = current->next;
}
if (current == NULL) {
current = createNode(arr[i]);
if (head == NULL) {
head = current;
} else {
current->next = head;
head = current;
}
}
}
}
// 打印链表
void printList(Node* node) {
while (node != NULL) {
printf("%d ", node->data);
node = node->next;
}
printf("\n");
}
int main() {
int arr[] = {1, 2, 3, 2, 4, 5, 6, 4};
int n = sizeof(arr) / sizeof(arr[0]);
printf("原始数组: ");
printList(head); // 假设头指针初始为空
removeDuplicates(arr, n);
printf("去重后的数组: ");
printList(head); // 输出去重后的链表
return 0;
}
```
这段代码首先遍历数组,如果遇到的元素不在链表中,则添加到链表头部。这样就可以保证链表中的元素都是唯一的。
阅读全文
相关推荐
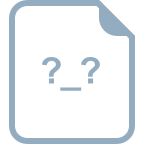



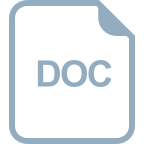

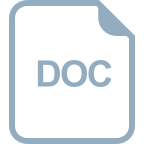
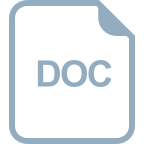
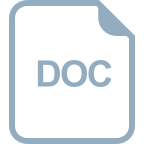

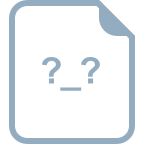
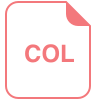




