编写程序实现使用Gin框架(或者不使用框架)在本地的8888端口启动GoWeb服务器,并且设置/index路径对应的处理函数是HandlerIndex
时间: 2024-03-25 08:35:19 浏览: 29
使用 Gin 框架编写的程序如下所示:
```go
package main
import (
"github.com/gin-gonic/gin"
"net/http"
)
func main() {
// 创建 Gin 实例
r := gin.Default()
// 设置 index 路径对应的处理函数
r.GET("/index", HandlerIndex)
// 启动服务器
err := r.Run(":8888")
if err != nil {
panic(err)
}
}
// 处理 index 路径的函数
func HandlerIndex(c *gin.Context) {
c.HTML(http.StatusOK, "index.html", gin.H{
"title": "Hello, Gin!",
})
}
```
在上面的代码中,我们首先创建了一个 Gin 实例,并使用 `r.GET` 函数将 `/index` 路径与 `HandlerIndex` 函数绑定。`HandlerIndex` 函数会将一个 HTML 模板渲染成 HTML 页面并返回给客户端。最后,我们使用 `r.Run` 函数启动服务器监听 8888 端口。
如果不使用 Gin 框架,可以使用 Go 标准库来实现:
```go
package main
import (
"net/http"
)
func main() {
// 设置 index 路径对应的处理函数
http.HandleFunc("/index", HandlerIndex)
// 启动服务器
err := http.ListenAndServe(":8888", nil)
if err != nil {
panic(err)
}
}
// 处理 index 路径的函数
func HandlerIndex(w http.ResponseWriter, r *http.Request) {
w.Header().Set("Content-Type", "text/html")
w.Write([]byte(`
<!DOCTYPE html>
<html>
<head>
<title>Hello, Go!</title>
</head>
<body>
<h1>Hello, Go!</h1>
</body>
</html>
`))
}
```
在上面的代码中,我们使用 `http.HandleFunc` 函数将 `/index` 路径与 `HandlerIndex` 函数绑定。`HandlerIndex` 函数直接将 HTML 页面写入响应体。最后,我们使用 `http.ListenAndServe` 函数启动服务器监听 8888 端口。
相关推荐
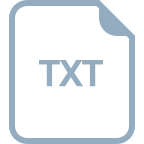
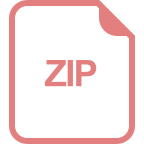
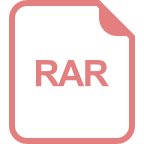














