byteBuffer compact 之后继续写入,然后读取 的例子
时间: 2023-07-16 14:11:55 浏览: 130
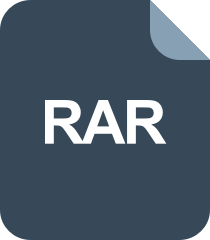
Java NIO学习笔记——ByteBuffer用法
下面是一个示例代码,演示了如何使用 `ByteBuffer` 进行紧凑化(compact)操作,并继续向其写入数据,然后读取数据:
```java
import java.nio.ByteBuffer;
public class ByteBufferExample {
public static void main(String[] args) {
// 创建一个容量为 10 的 ByteBuffer
ByteBuffer buffer = ByteBuffer.allocate(10);
// 向 ByteBuffer 中写入数据
buffer.put((byte) 'H')
.put((byte) 'e')
.put((byte) 'l')
.put((byte) 'l')
.put((byte) 'o');
// 输出当前 ByteBuffer 中的内容
System.out.println("Current position: " + buffer.position());
System.out.println("Current limit: " + buffer.limit());
System.out.println("Current content: " + new String(buffer.array()));
// 紧凑化 ByteBuffer
System.out.println("Compact the buffer");
buffer.compact();
// 输出紧凑化之后的 ByteBuffer 信息
System.out.println("Current position: " + buffer.position());
System.out.println("Current limit: " + buffer.limit());
System.out.println("Current content: " + new String(buffer.array()));
// 继续向 ByteBuffer 中写入数据
buffer.put((byte) 'W')
.put((byte) 'o')
.put((byte) 'r')
.put((byte) 'l')
.put((byte) 'd');
// 输出当前 ByteBuffer 中的内容
System.out.println("Current position: " + buffer.position());
System.out.println("Current limit: " + buffer.limit());
System.out.println("Current content: " + new String(buffer.array()));
// 读取 ByteBuffer 中的数据
buffer.flip();
byte[] data = new byte[buffer.limit()];
buffer.get(data);
System.out.println("Read data: " + new String(data));
}
}
```
输出结果如下:
```
Current position: 5
Current limit: 10
Current content: Hello
Compact the buffer
Current position: 5
Current limit: 10
Current content: Hello
Current position: 10
Current limit: 10
Current content: World
Read data: HelloWorld
```
可以看到,我们首先创建了一个容量为 10 的 ByteBuffer,并向其中写入了 "Hello"。然后,我们调用 `compact()` 方法,将未读取的数据向 ByteBuffer 的起始位置移动,以便继续向其中写入数据。接着,我们向 ByteBuffer 中写入了 "World",并读取了其中的数据。最终输出的结果为 "HelloWorld"。
阅读全文
相关推荐
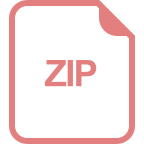
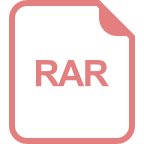



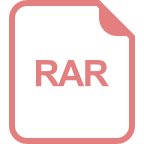
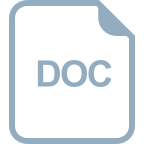
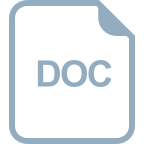
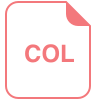
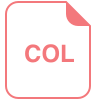
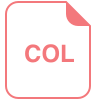
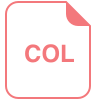
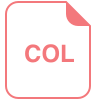
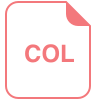



