编写一个算法,在头节点为h的单链表中,把值为b的结点插入到值为a的结点之前,若不存在a,则把结点s插入到值为a的结点之前,若不存在a,则把结点s插入到表尾 c语言实现
时间: 2024-09-29 11:08:49 浏览: 38
为了在头节点为 `h` 的单链表中插入节点,我们首先需要遍历链表找到值为 `a` 的节点,然后根据要求进行插入。这里是一个C语言的示例代码来完成这个任务:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构
struct Node {
int data;
struct Node* next;
};
// 插入节点到链表
void insertNode(struct Node** head, int a, int b, int s) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = b;
// 遍历链表查找值为a的节点
struct Node* current = *head;
while (current != NULL && current->data != a) {
current = current->next;
}
// 根据情况插入
if (current != NULL) { // 存在a节点
newNode->next = current->next;
current->next = newNode;
} else { // 不存在a节点,可能是第一个节点或找不到
if (*head == NULL || (*head)->data == a) { // a不存在于链表中,将s插入表首
newNode->next = *head;
*head = newNode;
} else {
newNode->next = NULL;
// 若存在其他非a节点,将s插入其后作为表尾
while (current->next != NULL && current->next->data != a) {
current = current->next;
}
current->next = newNode;
}
}
}
// 打印链表
void printList(struct Node* head) {
struct Node* current = head;
while (current != NULL) {
printf("%d -> ", current->data);
current = current->next;
}
printf("NULL\n");
}
int main() {
struct Node* h = (struct Node*)malloc(sizeof(struct Node));
h->data = 1; // 假设头节点h的数据
h->next = NULL;
insertNode(&h, 2, 3, 4); // 插入示例
printList(h); // 输出插入后的链表
return 0;
}
```
在这个例子中,`insertNode` 函数负责插入操作,`printList` 函数用于查看链表状态。在`main`函数中,你需要根据实际需求设置头节点 `h` 和要插入的值。
阅读全文
相关推荐





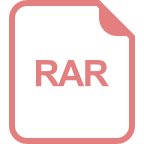


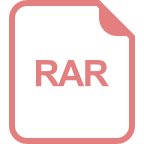


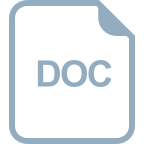




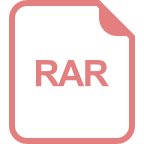
