用python将图片转化为16色图
时间: 2023-02-09 13:53:11 浏览: 186
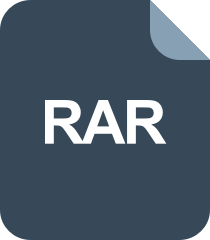
16进制图片转换

可以使用 Python 库 Pillow 来将图片转化为 16 色图。首先,需要安装 Pillow:
```python
pip install pillow
```
然后,可以使用以下代码打开图片并转化为 16 色:
```python
from PIL import Image
# Open image
im = Image.open("image.jpg")
# Convert to 16-color palette
im = im.convert("P", palette=Image.ADAPTIVE, colors=16)
# Save as a new image
im.save("16color.png")
```
上述代码默认使用"adaptive"调色板模式,也可以使用"Web"模式,调整为"palette=Image.WEB,colors=16"
如果要将图像转换为更少的颜色,可以将“colors”参数设置为更小的值,如 2、4 或 8。
阅读全文
相关推荐
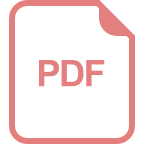
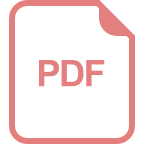
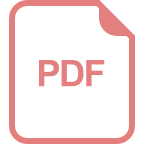
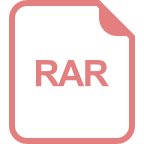
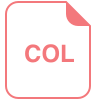

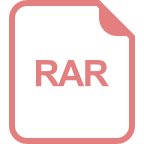
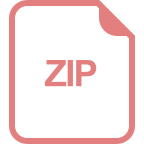
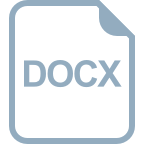
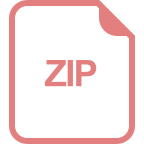
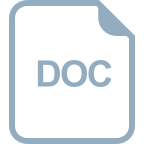
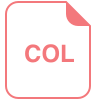
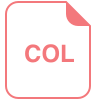
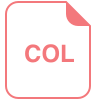
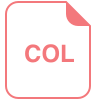

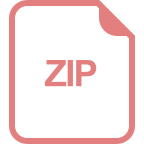