使用java实现解压字节字符串
时间: 2023-07-30 09:07:10 浏览: 106
在Java中可以使用 `java.util.zip` 包提供的类来解压缩字节数组。具体的实现步骤如下:
1. 创建一个 `Inflater` 对象。
```
Inflater inflater = new Inflater();
```
2. 为 `Inflater` 对象提供需要解压缩的数据,并指定解压缩数据的起始位置和长度。
```
inflater.setInput(compressedData, start, length);
```
3. 创建一个缓冲区,用于存储解压缩后的数据。
```
byte[] buffer = new byte[1024];
```
4. 循环读取解压缩后的数据,将读取到的数据写入缓冲区中。
```
int outputLength = inflater.inflate(buffer);
```
5. 关闭 `Inflater` 对象,释放资源。
```
inflater.end();
```
完整的代码示例如下:
```
import java.util.zip.Inflater;
public class Uncompressor {
public static byte[] uncompress(byte[] compressedData, int start, int length) throws Exception {
Inflater inflater = new Inflater();
inflater.setInput(compressedData, start, length);
byte[] buffer = new byte[1024];
int outputLength = 0;
ByteArrayOutputStream outputStream = new ByteArrayOutputStream();
try {
while (!inflater.finished()) {
outputLength = inflater.inflate(buffer);
outputStream.write(buffer, 0, outputLength);
}
} catch (DataFormatException e) {
throw new Exception("Failed to decompress data", e);
} finally {
inflater.end();
}
return outputStream.toByteArray();
}
}
```
调用示例:
```
byte[] compressedData = ... // 压缩后的字节数组
byte[] uncompressedData = Uncompressor.uncompress(compressedData, 0, compressedData.length);
```
其中 `compressedData` 为需要解压缩的字节数组,`start` 为解压缩数据的起始位置,`length` 为解压缩数据的长度。解压后的字节数组存储在 `uncompressedData` 中。
阅读全文
相关推荐





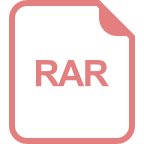
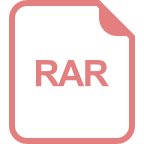
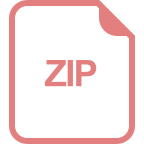
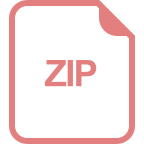
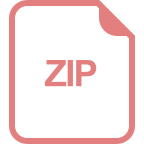
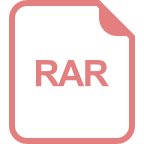
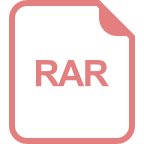
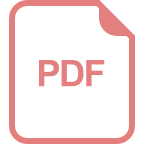
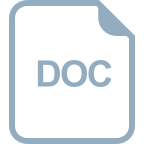
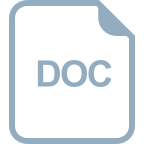
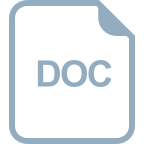