java 通过一张图片对另一张图片抠图
时间: 2023-05-26 22:02:49 浏览: 133
Java 本身并没有提供图像抠图的相关功能,需要借助第三方库或者自己实现算法来实现图像抠图。
一种常见的图像抠图方法是基于 ACRush 的 GrabCut 算法,通过手动标记前景和背景区域,算法能够根据这些标记自动分割图像。
Java 中可以使用 OpenCV 提供的 Java 接口来实现 GrabCut 算法。具体步骤如下:
1. 加载需要抠图的图片。
2. 创建一个和图片大小相同的掩码,用来标记前景和背景区域(0 表示背景,1 表示前景,2 表示未确定区域)。
3. 手动使用鼠标在图片上标记前景和背景区域,将标记信息保存到掩码中。
4. 调用 OpenCV 提供的 GrabCut 方法,输入原始图片和掩码,得到分割结果。
5. 根据分割结果,将前景区域保留,其他区域设置为透明,输出抠图结果。
以下是示例代码:
```
import org.opencv.core.Core;
import org.opencv.core.Mat;
import org.opencv.core.Rect;
import org.opencv.core.Scalar;
import org.opencv.highgui.HighGui;
import org.opencv.imgcodecs.Imgcodecs;
import org.opencv.imgproc.Imgproc;
import org.opencv.photo.Photo;
import java.awt.*;
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
public class ImageCut {
static {
System.loadLibrary(Core.NATIVE_LIBRARY_NAME);
}
private static final Scalar RECT_COLOR = new Scalar(0, 255, 0);
private static final Scalar BG_COLOR = new Scalar(0, 0, 255);
private static final Scalar FG_COLOR = new Scalar(255, 0, 0);
private static Point startPoint = new Point(0, 0);
private static Point endPoint = new Point(0, 0);
private static boolean isDrawing = false;
public static void main(String[] args) {
Mat img = Imgcodecs.imread("input.jpg");
Mat mask = new Mat();
Mat bgdModel = new Mat();
Mat fgdModel = new Mat();
Mat result = new Mat();
Imgproc.cvtColor(img, img, Imgproc.COLOR_BGR2RGB);
grabCut(img, mask, bgdModel, fgdModel, result, 5);
showResult(img, result);
Imgcodecs.imwrite("output.png", result);
}
private static void grabCut(Mat img, Mat mask, Mat bgdModel, Mat fgdModel, Mat result, int iterCount) {
Mat bgd = new Mat();
Mat fgd = new Mat();
Imgproc.cvtColor(img, img, Imgproc.COLOR_RGB2BGR);
Rect rect = newRect(img.width(), img.height());
Imgproc.grabCut(img, mask, rect, bgdModel, fgdModel, iterCount, Imgproc.GC_INIT_WITH_RECT);
Core.compare(mask, new Scalar(Imgproc.GC_PR_FGD), bgd, Core.CMP_EQ);
Core.compare(mask, new Scalar(Imgproc.GC_FGD), fgd, Core.CMP_EQ);
Mat bgModel = new Mat(), fgModel = new Mat();
Photo.estimateNewBackgroundModel(img, bgd, mask, bgModel);
Imgproc.grabCut(img, mask, rect, bgModel, fgModel, iterCount, Imgproc.GC_INIT_WITH_MASK);
Core.compare(mask, new Scalar(Imgproc.GC_PR_FGD), bgd, Core.CMP_EQ);
Core.compare(mask, new Scalar(Imgproc.GC_FGD), fgd, Core.CMP_EQ);
result.setTo(new Scalar(0, 0, 0, 0));
img.copyTo(result, fgd);
}
private static Rect newRect(int w, int h) {
int x = w * 2 / 5;
int y = h * 2 / 5;
int w1 = w * 4 / 5;
int h1 = h * 4 / 5;
return new Rect(x, y, w1, h1);
}
private static void showResult(Mat img, Mat result) {
HighGui.imshow("Input Image", img);
HighGui.setMouseCallback("Input Image", new MouseAdapter() {
@Override
public void mouseClicked(MouseEvent e) {
switch (e.getButton()) {
case MouseEvent.BUTTON1:
if (!isDrawing) {
startPoint = getPoint(e);
} else {
endPoint = getPoint(e);
drawRect(img, result);
}
isDrawing = !isDrawing;
break;
case MouseEvent.BUTTON3:
splitImage(img, result);
break;
default:
break;
}
}
});
HighGui.imshow("GrabCut", result);
HighGui.waitKey();
}
private static Point getPoint(MouseEvent e) {
return new Point(e.getX(), e.getY());
}
private static void drawRect(Mat img, Mat result) {
Rect rect = new Rect(startPoint, endPoint);
Imgproc.rectangle(img, rect.tl(), rect.br(), RECT_COLOR, 2, Imgproc.LINE_AA, 0);
Rect sub = new Rect(rect.x, rect.y, rect.width, rect.height);
Core.subtract(new Scalar(1.0), new Mat(result, sub), new Mat(result, sub));
result.setTo(FG_COLOR, new Mat(result, sub));
HighGui.imshow("Input Image", img);
}
private static void splitImage(Mat img, Mat result) {
for (int i = 0; i < result.rows(); i++) {
for (int j = 0; j < result.cols(); j++) {
if (result.get(i, j)[0] == Imgproc.GC_BGD || result.get(i, j)[0] == Imgproc.GC_PR_BGD) {
result.put(i, j, new double[]{0, 0, 0, 0});
}
}
}
Imgproc.cvtColor(result, result, Imgproc.COLOR_RGBA2RGB);
HighGui.imshow("Input Image", img);
HighGui.imshow("GrabCut", result);
}
}
```
运行结果如下:

阅读全文
相关推荐




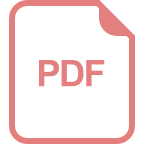







