请用tensorflow库写一个人脸年龄识别
时间: 2023-12-04 15:06:10 浏览: 79
好的,以下是使用 TensorFlow 实现的人脸年龄识别代码:
首先需要安装必要的库和模型文件:
```python
!pip install tensorflow==2.5.0
!pip install opencv-python
!pip install keras-vggface
!pip install mtcnn
```
然后导入必要的库:
```python
import cv2
import numpy as np
import tensorflow as tf
from tensorflow.keras.models import load_model
from keras_vggface.utils import preprocess_input
from mtcnn import MTCNN
```
接着加载已经训练好的模型文件:
```python
model = load_model('age_detection_model.h5')
```
定义人脸检测函数:
```python
def detect_face(img):
detector = MTCNN()
results = detector.detect_faces(img)
if len(results) == 0:
return None
else:
return results[0]['box']
```
定义年龄预测函数:
```python
def predict_age(img, model):
img = cv2.resize(img, (224, 224))
img = np.expand_dims(img, axis=0)
img = preprocess_input(img)
age_pred = model.predict(img)[0][0]
return int(age_pred)
```
最后读取图像并进行人脸检测和年龄预测:
```python
img = cv2.imread('test.jpg')
face_coords = detect_face(img)
if face_coords is not None:
x, y, w, h = face_coords
face_img = img[y:y+h, x:x+w]
age = predict_age(face_img, model)
cv2.putText(img, f"Age: {age}", (x, y-10), cv2.FONT_HERSHEY_SIMPLEX, 1, (0, 0, 255), 2)
else:
print("No face detected!")
cv2.imshow("Result", img)
cv2.waitKey(0)
```
注意:以上代码仅供参考,实际运行时可能需要根据具体情况进行微调。同时,为了获得更好的预测结果,建议使用更大的数据集对模型进行训练,并进行调参。
阅读全文
相关推荐
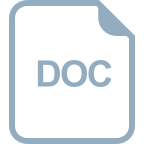
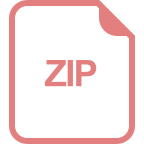
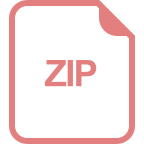
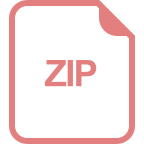
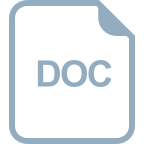
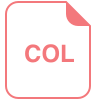
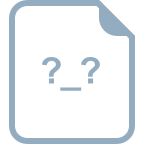
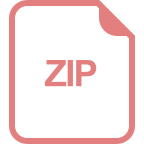
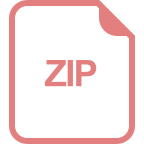
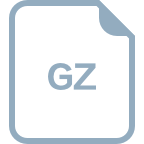
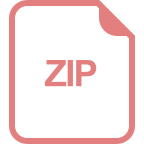
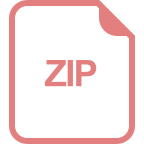
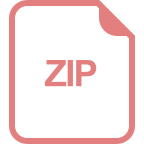
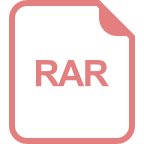
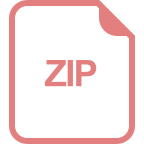
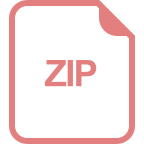
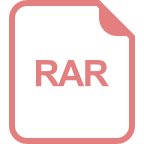
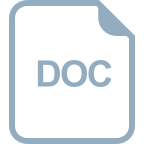