spring security 用法
时间: 2023-07-04 13:16:57 浏览: 50
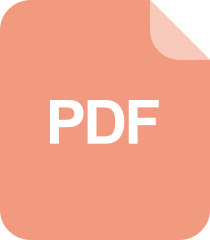
spring security 一步一步教你使用

Spring Security 是一个基于 Spring 框架的安全框架,用于实现应用程序的身份认证和授权管理。Spring Security 提供了多种认证和授权方式,包括基于表单、HTTP 基本认证、OAuth2、JWT 等,可以灵活地满足各种应用程序的需求。
以下是 Spring Security 的基本用法:
1. 添加 Spring Security 依赖
在 Maven 项目中,可以通过在 pom.xml 文件中添加以下依赖来引入 Spring Security:
```xml
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-web</artifactId>
<version>5.4.1</version>
</dependency>
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-config</artifactId>
<version>5.4.1</version>
</dependency>
```
2. 配置 Spring Security
在 Spring Boot 应用程序中,可以通过在 application.yml 或 application.properties 文件中添加以下配置来启用 Spring Security:
```yaml
spring:
security:
user:
name: user
password: password
```
上述配置指定了一个默认的用户名和密码,用于测试和演示。
3. 添加安全配置
可以通过创建一个继承自 WebSecurityConfigurerAdapter 的配置类来添加安全配置,并重写其中的 configure 方法来配置身份认证和授权管理。例如:
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN")
.antMatchers("/user/**").hasRole("USER")
.anyRequest().authenticated()
.and()
.formLogin()
.and()
.logout()
.logoutUrl("/logout")
.logoutSuccessUrl("/")
.invalidateHttpSession(true)
.deleteCookies("JSESSIONID");
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("user").password("{noop}password").roles("USER")
.and()
.withUser("admin").password("{noop}password").roles("ADMIN");
}
}
```
上述配置指定了两个用户,分别具有 USER 和 ADMIN 角色,其中 ADMIN 用户可以访问 /admin/** 的 URL,而 USER 用户可以访问 /user/** 的 URL。其他未指定的 URL 需要进行身份认证才能访问。同时,还配置了表单登录和退出登录的处理。
4. 添加安全注解
可以通过在控制器方法上添加 @PreAuthorize 或 @Secured 注解来指定访问该方法需要的角色或权限。例如:
```java
@RestController
@RequestMapping("/admin")
public class AdminController {
@PreAuthorize("hasRole('ADMIN')")
@GetMapping("/users")
public List<User> getAllUsers() {
return userService.getAllUsers();
}
}
```
上述代码指定了 getAllUsers 方法需要 ADMIN 角色才能访问。
以上是 Spring Security 的基本用法,具体的身份认证和授权管理方式还有很多,需要根据实际情况进行选择和配置。
阅读全文
相关推荐

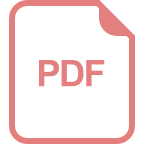
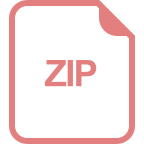
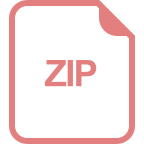



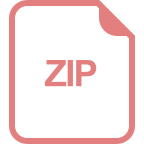
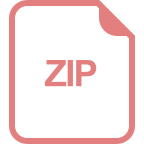
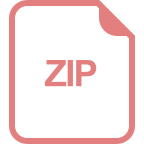
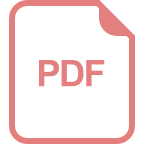
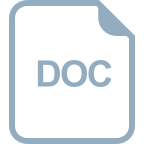

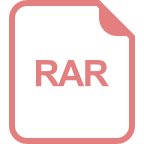
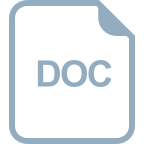
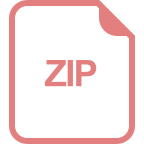
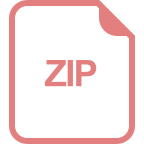