java excel替换字符串_java操作excel 替换指定字符串 并生成新的文件
时间: 2023-07-06 15:27:04 浏览: 49
你可以使用 Apache POI 库来操作 Excel 文件,实现替换指定字符串并生成新的文件。
以下是一个简单的示例代码:
```java
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class ExcelReplacer {
public static void main(String[] args) throws IOException {
// 读取 Excel 文件
File file = new File("path/to/your/excel/file.xlsx");
FileInputStream inputStream = new FileInputStream(file);
Workbook workbook = new XSSFWorkbook(inputStream);
// 获取第一个 Sheet
Sheet sheet = workbook.getSheetAt(0);
// 遍历每一行
for (Row row : sheet) {
// 遍历每一列
for (Cell cell : row) {
// 如果单元格的值包含指定的字符串,就替换为新的字符串
if (cell.getCellType() == Cell.CELL_TYPE_STRING && cell.getStringCellValue().contains("oldValue")) {
String oldValue = cell.getStringCellValue();
String newValue = oldValue.replace("oldValue", "newValue");
cell.setCellValue(newValue);
}
}
}
// 生成新的 Excel 文件
FileOutputStream outputStream = new FileOutputStream("path/to/new/excel/file.xlsx");
workbook.write(outputStream);
workbook.close();
}
}
```
在这个示例中,我们首先读取 Excel 文件,然后获取第一个 Sheet,并遍历每一行和每一列,查找包含指定字符串的单元格,将其替换为新的字符串。最后,我们将修改后的 Excel 文件生成到指定的路径。
相关推荐
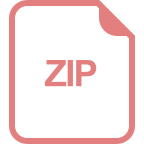
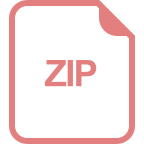
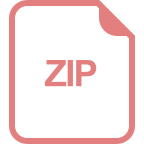














