三路抢答器c语言程序
时间: 2023-09-27 08:06:05 浏览: 134
以下是一个三路抢答器的 C 语言程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <unistd.h>
#define NUM_THREADS 3
int current = 0;
pthread_mutex_t mutex;
pthread_cond_t cond_var[NUM_THREADS];
void *thread_func(void *id_ptr) {
int id = *((int*)id_ptr);
while (1) {
pthread_mutex_lock(&mutex);
while (id != current) {
pthread_cond_wait(&cond_var[id], &mutex);
}
printf("Thread %d got the lock!\n", id);
sleep(1);
current = (current + 1) % NUM_THREADS;
pthread_cond_signal(&cond_var[current]);
pthread_mutex_unlock(&mutex);
}
return NULL;
}
int main(int argc, char *argv[]) {
pthread_t threads[NUM_THREADS];
int thread_ids[NUM_THREADS];
pthread_mutex_init(&mutex, NULL);
for (int i = 0; i < NUM_THREADS; i++) {
pthread_cond_init(&cond_var[i], NULL);
thread_ids[i] = i;
pthread_create(&threads[i], NULL, thread_func, &thread_ids[i]);
}
pthread_join(threads[0], NULL);
return 0;
}
```
该程序使用了 pthread 库来创建了三个线程,每个线程都在一个无限循环中等待抢答器的锁。当某个线程获得锁时,它会打印一条消息,并等待一秒钟,然后释放锁,并通知下一个线程可以获取锁了。每个线程都有一个 ID,用于确定它在抢答器中的顺序。程序中使用了条件变量来实现等待和通知的功能。
阅读全文
相关推荐
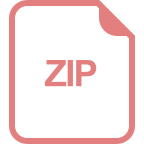
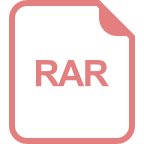
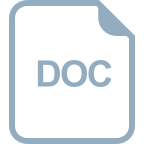
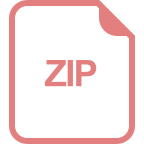
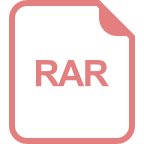
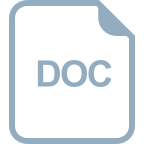
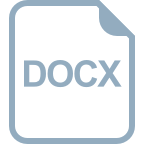
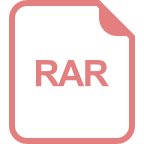
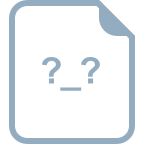
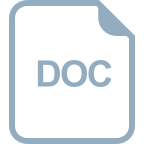
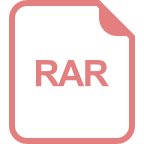
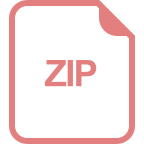
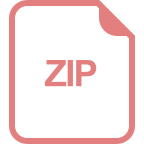
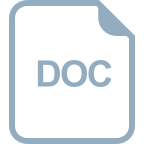
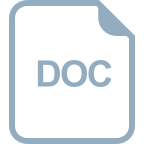
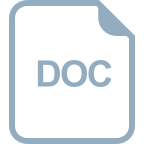