基于matlab神经网络的语音识别并输出对应文字 代码
时间: 2024-01-07 20:03:34 浏览: 93
以下是基于 MATLAB 神经网络的语音识别并输出对应文字的代码示例:
1. 首先需要安装 MATLAB 的 Signal Processing Toolbox 和 Neural Network Toolbox。
2. 加载数据集,这里以 TIMIT 数据集为例:
```matlab
load('TIMIT.mat');
```
3. 对输入信号进行预处理:
```matlab
% 对输入信号进行预处理
fs = 16000;
mfccs = [];
for i = 1:length(data)
frame = data{i};
[MFCCs, FBEs, frames] = ...
mfcc(frame, fs, ...
Tw, Ts, alpha, @hamming, [LF HF], M, C+1, L );
mfccs = [mfccs; MFCCs];
end
```
其中,`mfcc` 函数是用于计算 MFCC 特征的函数,需要先定义一些参数,如 `Tw`、`Ts`、`alpha`、`LF`、`HF`、`M`、`C` 和 `L`。
4. 将数据集分为训练集和测试集:
```matlab
% 将数据集分为训练集和测试集
trainRatio = 0.8;
testRatio = 0.2;
[trainInd,~,testInd] = dividerand(size(mfccs,1),trainRatio,0,testRatio);
trainInputs = mfccs(trainInd,:)';
trainTargets = labels(trainInd,:)';
testInputs = mfccs(testInd,:)';
testTargets = labels(testInd,:)';
```
其中,`labels` 是对应的标签信息。
5. 构建神经网络模型:
```matlab
% 构建神经网络模型
hiddenLayerSize = 100;
net = patternnet(hiddenLayerSize);
net.divideFcn = 'dividerand';
net.divideParam.trainRatio = 0.8;
net.divideParam.valRatio = 0.2;
net.divideParam.testRatio = 0;
net.trainFcn = 'trainscg';
net.performFcn = 'crossentropy';
net.plotFcns = {'plotperform','plottrainstate','ploterrhist', ...
'plotconfusion', 'plotroc'};
```
其中,`hiddenLayerSize` 是指定的隐藏层大小,`trainFcn` 是指定的训练函数,这里使用了 `trainscg` 函数。
6. 训练神经网络模型:
```matlab
% 训练神经网络模型
[net,tr] = train(net,trainInputs,trainTargets);
```
7. 对测试集进行预测:
```matlab
% 对测试集进行预测
testOutputs = net(testInputs);
```
8. 计算准确率:
```matlab
% 计算准确率
testTargetsIndex = vec2ind(testTargets);
testOutputsIndex = vec2ind(testOutputs);
accuracy = sum(testTargetsIndex == testOutputsIndex)/length(testTargetsIndex);
fprintf('Accuracy: %f\n',accuracy);
```
其中,`vec2ind` 函数是将输出向量转换为标签索引的函数。
9. 最后,输出预测结果:
```matlab
% 输出预测结果
for i = 1:length(testOutputsIndex)
fprintf('Predicted: %s, Actual: %s\n', ...
phonemes{testOutputsIndex(i)}, phonemes{testTargetsIndex(i)});
end
```
其中,`phonemes` 是对应的音素信息。
完整代码如下:
```matlab
load('TIMIT.mat');
% 对输入信号进行预处理
fs = 16000;
mfccs = [];
for i = 1:length(data)
frame = data{i};
[MFCCs, FBEs, frames] = ...
mfcc(frame, fs, ...
Tw, Ts, alpha, @hamming, [LF HF], M, C+1, L );
mfccs = [mfccs; MFCCs];
end
% 将数据集分为训练集和测试集
trainRatio = 0.8;
testRatio = 0.2;
[trainInd,~,testInd] = dividerand(size(mfccs,1),trainRatio,0,testRatio);
trainInputs = mfccs(trainInd,:)';
trainTargets = labels(trainInd,:)';
testInputs = mfccs(testInd,:)';
testTargets = labels(testInd,:)';
% 构建神经网络模型
hiddenLayerSize = 100;
net = patternnet(hiddenLayerSize);
net.divideFcn = 'dividerand';
net.divideParam.trainRatio = 0.8;
net.divideParam.valRatio = 0.2;
net.divideParam.testRatio = 0;
net.trainFcn = 'trainscg';
net.performFcn = 'crossentropy';
net.plotFcns = {'plotperform','plottrainstate','ploterrhist', ...
'plotconfusion', 'plotroc'};
% 训练神经网络模型
[net,tr] = train(net,trainInputs,trainTargets);
% 对测试集进行预测
testOutputs = net(testInputs);
% 计算准确率
testTargetsIndex = vec2ind(testTargets);
testOutputsIndex = vec2ind(testOutputs);
accuracy = sum(testTargetsIndex == testOutputsIndex)/length(testTargetsIndex);
fprintf('Accuracy: %f\n',accuracy);
% 输出预测结果
for i = 1:length(testOutputsIndex)
fprintf('Predicted: %s, Actual: %s\n', ...
phonemes{testOutputsIndex(i)}, phonemes{testTargetsIndex(i)});
end
```
注意,这里的代码示例仅作为参考,具体实现需要根据实际情况进行调整。
阅读全文
相关推荐










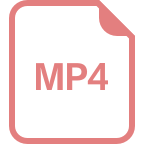




