使用java1.8和spark获取hbase表中三个字段的全量数据后以"\t"连接后保存到hdfss上
时间: 2024-02-11 13:07:10 浏览: 186
好的,我可以为您提供一些相关的Java代码实现,具体如下:
```java
// 导入相关的依赖包
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.FileSystem;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.hbase.Cell;
import org.apache.hadoop.hbase.HBaseConfiguration;
import org.apache.hadoop.hbase.TableName;
import org.apache.hadoop.hbase.client.Connection;
import org.apache.hadoop.hbase.client.ConnectionFactory;
import org.apache.hadoop.hbase.client.Get;
import org.apache.hadoop.hbase.client.Result;
import org.apache.hadoop.hbase.client.Table;
import org.apache.hadoop.hbase.util.Bytes;
import org.apache.spark.SparkConf;
import org.apache.spark.api.java.JavaRDD;
import org.apache.spark.api.java.JavaSparkContext;
import java.io.IOException;
import java.net.URI;
import java.util.ArrayList;
import java.util.List;
public class HBaseToHDFSSave {
public static void main(String[] args) throws IOException {
// HBase 配置
Configuration hbaseConf = HBaseConfiguration.create();
hbaseConf.set("hbase.zookeeper.quorum", "localhost");
hbaseConf.set("hbase.zookeeper.property.clientPort", "2181");
Connection hbaseConn = ConnectionFactory.createConnection(hbaseConf);
// Spark 配置
SparkConf sparkConf = new SparkConf().setAppName("HBaseToHDFSSave").setMaster("local[*]");
JavaSparkContext sparkContext = new JavaSparkContext(sparkConf);
// 从 HBase 表中读取数据
String tableName = "table_name";
Table table = hbaseConn.getTable(TableName.valueOf(tableName));
Get get = new Get(Bytes.toBytes("row_key"));
Result result = table.get(get);
// 将结果保存到 List 中
List<String> resultList = new ArrayList<>();
for (Cell cell : result.listCells()) {
String rowKey = Bytes.toString(cell.getRowArray(), cell.getRowOffset(), cell.getRowLength());
String columnFamily = Bytes.toString(cell.getFamilyArray(), cell.getFamilyOffset(), cell.getFamilyLength());
String qualifier = Bytes.toString(cell.getQualifierArray(), cell.getQualifierOffset(), cell.getQualifierLength());
String value = Bytes.toString(cell.getValueArray(), cell.getValueOffset(), cell.getValueLength());
String rowValue = String.join("\t", rowKey, columnFamily, qualifier, value);
resultList.add(rowValue);
}
// 将 List 转换为 RDD 并保存到 HDFS
JavaRDD<String> resultRDD = sparkContext.parallelize(resultList);
FileSystem fileSystem = FileSystem.get(URI.create("hdfs://localhost:9000/"), new Configuration());
Path outputPath = new Path("/output/path");
if (fileSystem.exists(outputPath)) {
fileSystem.delete(outputPath, true);
}
resultRDD.saveAsTextFile(outputPath.toString());
// 关闭资源
table.close();
hbaseConn.close();
sparkContext.close();
}
}
```
这是一个简单的 Java 程序,可以通过 Spark 从 HBase 表中读取数据,并将结果保存到 HDFS 上。您需要将相关的配置参数和表名、行键、列族、列限定符等信息替换为实际的值,同时需要指定 HDFS 的输出路径。
阅读全文
相关推荐
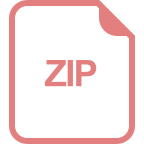
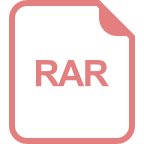
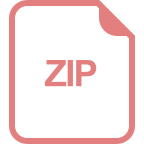
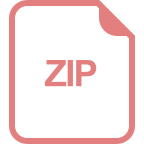
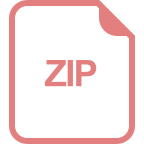
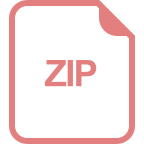
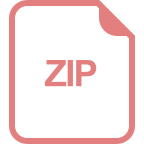
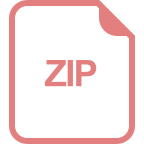
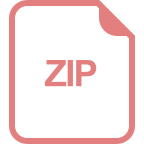
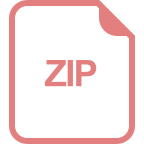
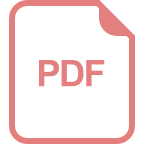
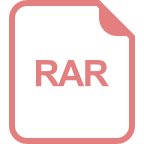
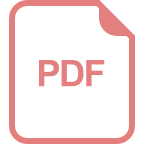
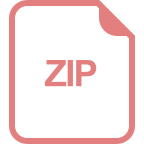
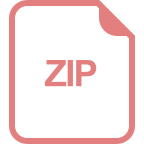
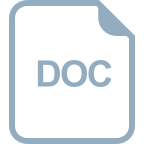
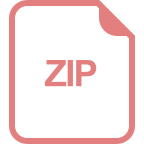
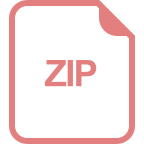