ConcurrentSkipListMap 遍历
时间: 2023-06-21 20:04:52 浏览: 69
ConcurrentSkipListMap 是一个线程安全的有序映射表,它的内部结构是基于跳表实现的。遍历 ConcurrentSkipListMap 有如下几种方式:
1. 迭代器遍历:使用迭代器对 ConcurrentSkipListMap 进行遍历,代码示例如下:
```
ConcurrentSkipListMap<String, Integer> map = new ConcurrentSkipListMap<>();
// 向 map 中添加元素
Iterator<Map.Entry<String, Integer>> iterator = map.entrySet().iterator();
while (iterator.hasNext()) {
Map.Entry<String, Integer> entry = iterator.next();
String key = entry.getKey();
Integer value = entry.getValue();
// 处理 key 和 value
}
```
2. forEach 遍历:使用 forEach 方法对 ConcurrentSkipListMap 进行遍历,代码示例如下:
```
ConcurrentSkipListMap<String, Integer> map = new ConcurrentSkipListMap<>();
// 向 map 中添加元素
map.forEach((key, value) -> {
// 处理 key 和 value
});
```
3. 并发迭代器遍历:使用 ConcurrentHashMap.KeySetView 或者 ConcurrentHashMap.EntrySetView 的并发迭代器对 ConcurrentSkipListMap 进行遍历,代码示例如下:
```
ConcurrentSkipListMap<String, Integer> map = new ConcurrentSkipListMap<>();
// 向 map 中添加元素
ConcurrentHashMap.KeySetView<String, Boolean> keySetView = map.keySet();
Iterator<String> iterator = keySetView.iterator();
while (iterator.hasNext()) {
String key = iterator.next();
Integer value = map.get(key);
// 处理 key 和 value
}
// 或者
ConcurrentSkipListMap<String, Integer> map = new ConcurrentSkipListMap<>();
// 向 map 中添加元素
ConcurrentHashMap.EntrySetView<String, Integer> entrySetView = map.entrySet();
Iterator<Map.Entry<String, Integer>> iterator = entrySetView.iterator();
while (iterator.hasNext()) {
Map.Entry<String, Integer> entry = iterator.next();
String key = entry.getKey();
Integer value = entry.getValue();
// 处理 key 和 value
}
```
需要注意的是,在遍历 ConcurrentSkipListMap 时,由于它是有序的,因此迭代器遍历和 forEach 遍历会按照键的自然顺序进行遍历。而并发迭代器遍历则没有顺序保证,因此适用于并发环境下的场景。
相关推荐
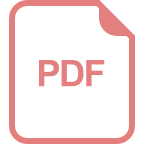
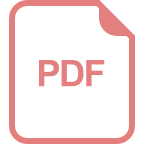
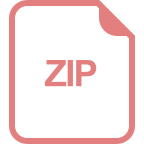
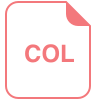













