python百度智能云图像识别文字 图形结合
时间: 2023-08-21 19:05:15 浏览: 67
百度智能云提供了丰富的图像识别API,包括OCR文字识别和图像识别等。可以使用Python的requests库和百度智能云提供的API接口进行图像识别。
具体步骤如下:
1. 在百度智能云控制台创建应用,获取API Key和Secret Key。
2. 安装requests库,使用API Key和Secret Key生成Access Token。
3. 使用Access Token和API接口对图像进行识别,获取识别结果。
示例代码如下:
```python
import requests
import base64
# 获取Access Token
def get_access_token(api_key, secret_key):
url = 'https://aip.baidubce.com/oauth/2.0/token'
params = {
'grant_type': 'client_credentials',
'client_id': api_key,
'client_secret': secret_key,
}
response = requests.post(url, params=params)
if response:
access_token = response.json()['access_token']
return access_token
else:
return None
# OCR文字识别
def ocr_text_recognition(image_path, access_token):
url = 'https://aip.baidubce.com/rest/2.0/ocr/v1/general_basic'
with open(image_path, 'rb') as f:
image_data = f.read()
image_base64 = base64.b64encode(image_data).decode('utf-8')
data = {'image': image_base64}
headers = {'Content-Type': 'application/x-www-form-urlencoded'}
params = {'access_token': access_token}
response = requests.post(url, params=params, headers=headers, data=data)
if response:
result = response.json()['words_result']
return result
else:
return None
# 物体识别
def object_detection(image_path, access_token):
url = 'https://aip.baidubce.com/rest/2.0/image-classify/v2/advanced_general'
with open(image_path, 'rb') as f:
image_data = f.read()
image_base64 = base64.b64encode(image_data).decode('utf-8')
data = {'image': image_base64}
headers = {'Content-Type': 'application/x-www-form-urlencoded'}
params = {'access_token': access_token}
response = requests.post(url, params=params, headers=headers, data=data)
if response:
result = response.json()['result']
return result
else:
return None
if __name__ == '__main__':
# 替换为自己的API Key和Secret Key
api_key = 'your_api_key'
secret_key = 'your_secret_key'
# 获取Access Token
access_token = get_access_token(api_key, secret_key)
# 图像识别
image_path = 'test.jpg'
text_result = ocr_text_recognition(image_path, access_token)
object_result = object_detection(image_path, access_token)
# 输出识别结果
print(text_result)
print(object_result)
```
以上代码演示了如何使用Python和百度智能云API实现OCR文字识别和物体识别。可以根据自己的需求修改API接口和参数,实现更多功能。
相关推荐
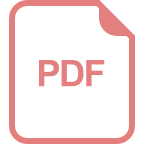
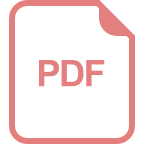














