使用opencv c++实现车道线检测
时间: 2023-11-04 09:42:08 浏览: 50
Hi! How can I help you with OpenCV and lane detection today?
这是一个关于如何使用OpenCV c实现车道线检测的问题。
Sure, I'd be happy to help you with that! Can you provide more details about what you're trying to accomplish and what you've tried so far?
相关问题
OpenCV C++实现车道线检测
OpenCV C++实现车道线检测的基本思路如下:
1. 读取图像并进行灰度化处理。
2. 对灰度图像进行高斯滤波,去除噪声。
3. 对滤波后的图像进行Canny边缘检测,得到边缘图像。
4. 对边缘图像进行霍夫变换,检测直线。
5. 对检测到的直线进行筛选和拟合,得到车道线。
下面是OpenCV C++实现车道线检测的代码示例:
```cpp
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace cv;
using namespace std;
int main()
{
// 读取图像
Mat src = imread("test.jpg");
// 灰度化处理
Mat gray;
cvtColor(src, gray, COLOR_BGR2GRAY);
// 高斯滤波
Mat blur;
GaussianBlur(gray, blur, Size(5, 5), 0);
// Canny边缘检测
Mat edges;
Canny(blur, edges, 50, 150);
// 霍夫变换检测直线
vector<Vec2f> lines;
HoughLines(edges, lines, 1, CV_PI / 180, 100);
// 筛选和拟合直线
vector<Vec4i> lanes;
for (size_t i = 0; i < lines.size(); i++)
{
float rho = lines[i][0], theta = lines[i][1];
if (theta < CV_PI / 4 || theta > 3 * CV_PI / 4)
{
Point pt1(rho / cos(theta), 0);
Point pt2((rho - edges.rows * sin(theta)) / cos(theta), edges.rows); lanes.push_back(Vec4i(pt1.x, pt1.y, pt2.x, pt2.y));
}
}
// 绘制车道线
Mat result = src.clone();
for (size_t i = 0; i < lanes.size(); i++)
{
line(result, Point(lanes[i][0], lanes[i][1]), Point(lanes[i][2], lanes[i][3]), Scalar(0, 0, 255), 3, LINE_AA);
}
// 显示结果
imshow("result", result);
waitKey(0);
return 0;
}
```
opencv c++实现车道线检测代码实现
以下是一个简单的基于OpenCV的C++实现车道线检测的代码示例:
```
#include <iostream>
#include <opencv2/opencv.hpp>
using namespace std;
using namespace cv;
int main()
{
// 读入图像
Mat img = imread("lane.jpg");
// 转换为灰度图像
Mat gray;
cvtColor(img, gray, COLOR_BGR2GRAY);
// 使用高斯滤波去除噪声
Mat blur;
GaussianBlur(gray, blur, Size(5, 5), 0);
// 使用Canny算子进行边缘检测
Mat edges;
Canny(blur, edges, 50, 150);
// 定义ROI(感兴趣区域)
Mat roi;
Point points[4] = {Point(0, img.rows), Point(img.cols / 2, img.rows / 2), Point(img.cols / 2, img.rows / 2), Point(img.cols, img.rows)};
Mat mask = Mat::zeros(img.size(), CV_8UC1);
fillConvexPoly(mask, points, 4, Scalar(255));
bitwise_and(edges, mask, roi);
// 使用霍夫变换进行直线检测
vector<Vec4i> lines;
HoughLinesP(roi, lines, 1, CV_PI / 180, 50, 50, 10);
// 绘制检测到的直线
for (size_t i = 0; i < lines.size(); i++)
{
line(img, Point(lines[i][0], lines[i][1]), Point(lines[i][2], lines[i][3]), Scalar(0, 0, 255), 2);
}
// 显示结果
imshow("Lane Detection", img);
waitKey(0);
return 0;
}
```
这个代码实现了以下步骤:
1. 读入图像,并将其转换为灰度图像。
2. 使用高斯滤波器去除噪声。
3. 使用Canny算子检测边缘。
4. 定义ROI(感兴趣区域),并应用到边缘图像上。
5. 使用霍夫变换进行直线检测。
6. 绘制检测到的直线。
7. 显示结果。
请注意,这只是一个基本示例,实际上车道线检测是一个相当复杂的任务,需要对多个因素进行考虑,包括照明条件、道路表面状况、车速等。此外,还可以使用更高级的技术,如深度学习等,来改进车道线检测的准确性和鲁棒性。
相关推荐
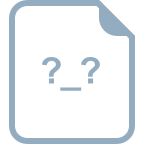
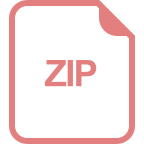
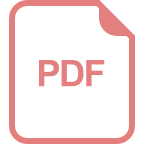









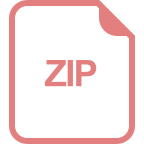
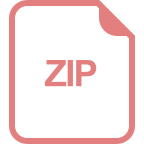
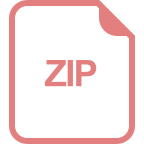