请用C语言编程菜单实现栈的基本操作,主要包括栈的创建、压栈栈和弹栈等基本操作。
时间: 2023-10-11 19:12:21 浏览: 149
好的,以下是使用C语言编写栈的基本操作的一个示例代码:
#include <stdio.h>
#include <stdlib.h>
#define STACK_SIZE 10 // 栈的最大容量
typedef int ElementType; // 定义栈中元素的类型
typedef struct Stack {
ElementType* data; // 栈数据存储区域的指针
int top; // 栈顶指针
int size; // 栈的当前大小
int capacity; // 栈的容量
} Stack;
void init_stack(Stack* s) {
s->data = (ElementType*)malloc(sizeof(ElementType) * STACK_SIZE);
s->top = -1;
s->size = 0;
s->capacity = STACK_SIZE;
}
void destroy_stack(Stack* s) {
free(s->data);
s->data = NULL;
s->top = -1;
s->size = 0;
s->capacity = 0;
}
int is_empty(Stack* s) {
return s->size == 0;
}
int is_full(Stack* s) {
return s->size == s->capacity;
}
int push(Stack* s, ElementType x) {
if (is_full(s)) {
printf("Stack is full, push failed\n");
return 0;
}
s->top++;
s->data[s->top] = x;
s->size++;
return 1;
}
int pop(Stack* s, ElementType* x) {
if (is_empty(s)) {
printf("Stack is empty, pop failed\n");
return 0;
}
*x = s->data[s->top];
s->top--;
s->size--;
return 1;
}
int main() {
Stack s;
init_stack(&s);
push(&s, 1);
push(&s, 2);
push(&s, 3);
ElementType x;
pop(&s, &x);
printf("%d\n", x);
pop(&s, &x);
printf("%d\n", x);
pop(&s, &x);
printf("%d\n", x);
destroy_stack(&s);
return 0;
}
阅读全文
相关推荐
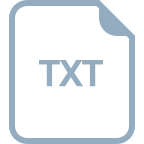


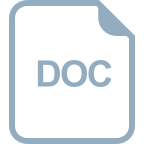
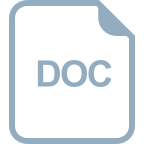
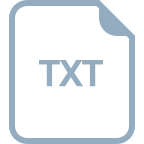
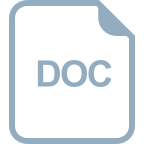
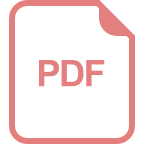
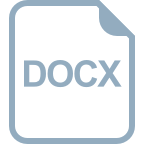
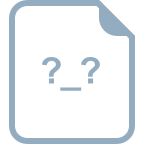
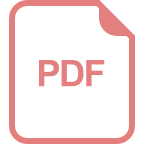
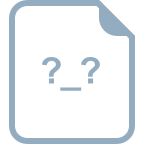
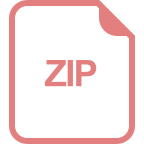
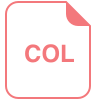