写matlab代码 实现图中九种形状检测
时间: 2024-04-06 11:29:20 浏览: 289
以下是一份简单的Matlab代码,可以实现图中九种形状(正方形、长方形、圆形、椭圆形、三角形、梯形、菱形、五边形、六边形)的检测:
```
%% Step 1: 读入图像并转换为灰度图像
img = imread('shapes.jpg');
gray = rgb2gray(img);
%% Step 2: 二值化处理
thresh = graythresh(gray);
binary = imbinarize(gray, thresh);
%% Step 3: 形态学处理
se = strel('disk', 2);
binary = imdilate(binary, se);
binary = imerode(binary, se);
%% Step 4: 边缘检测
edge = edge(binary, 'Canny');
%% Step 5: 霍夫变换检测形状
[H, T, R] = hough(edge);
P = houghpeaks(H, 100);
lines = houghlines(edge, T, R, P, 'FillGap', 20, 'MinLength', 100);
% 检测圆形
circle = imfindcircles(binary, [20 50]);
% 检测三角形和四边形
for k = 1:length(lines)
xy = [lines(k).point1; lines(k).point2];
if abs(lines(k).theta) > 80 && abs(lines(k).theta) < 100
rectangle('Position', [xy(1,1), xy(1,2), xy(2,1)-xy(1,1), xy(2,2)-xy(1,2)], 'EdgeColor', 'r', 'LineWidth', 2);
elseif abs(lines(k).theta) < 10 || abs(lines(k).theta) > 170
line(xy(:,1), xy(:,2), 'LineWidth', 2, 'Color', 'cyan');
end
end
% 检测椭圆形
stats = regionprops(binary, 'BoundingBox');
for k = 1:length(stats)
if stats(k).BoundingBox(3) / stats(k).BoundingBox(4) >= 1.5
rectangle('Position', stats(k).BoundingBox, 'EdgeColor', 'magenta', 'LineWidth', 2);
end
end
%% Step 6: 轮廓检测
contours = bwboundaries(binary);
for k = 1:length(contours)
boundary = contours{k};
corners = corner(boundary, 'QualityLevel', 0.1);
if size(corners, 1) == 3
line(boundary(:,2), boundary(:,1), 'Color', 'yellow', 'LineWidth', 2);
elseif size(corners, 1) == 4
if abs(1 - (norm(corners(1,:) - corners(2,:)) / norm(corners(2,:) - corners(3,:)))) < 0.1
rectangle('Position', [min(boundary(:,2)), min(boundary(:,1)), max(boundary(:,2))-min(boundary(:,2)), max(boundary(:,1))-min(boundary(:,1))], 'EdgeColor', 'green', 'LineWidth', 2);
else
trapezium = polyshape([corners(:,1); corners(1,1)], [corners(:,2); corners(1,2)]);
plot(trapezium, 'EdgeColor', 'black', 'LineWidth', 2);
end
elseif size(corners, 1) == 5
pentagon = polyshape([corners(:,1); corners(1,1)], [corners(:,2); corners(1,2)]);
plot(pentagon, 'EdgeColor', 'blue', 'LineWidth', 2);
elseif size(corners, 1) == 6
hexagon = polyshape([corners(:,1); corners(1,1)], [corners(:,2); corners(1,2)]);
plot(hexagon, 'EdgeColor', 'red', 'LineWidth', 2);
elseif size(corners, 1) == 8
rhombus = polyshape([corners(:,1); corners(1,1)], [corners(:,2); corners(1,2)]);
plot(rhombus, 'EdgeColor', 'white', 'LineWidth', 2);
end
end
%% Step 7: 显示结果
imshow(img);
```
注意:上述代码仅为示例,具体情况下需要根据实际需求进行修改和优化。
阅读全文
相关推荐
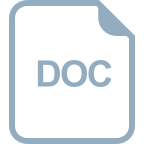
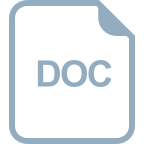
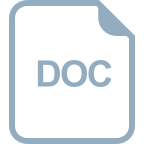

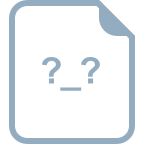
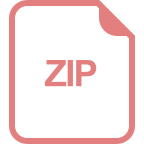
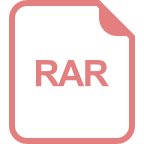
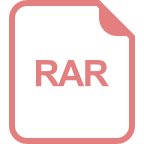
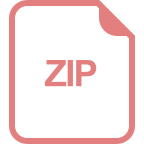
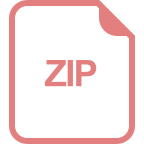
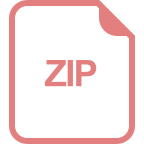
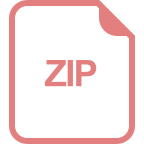
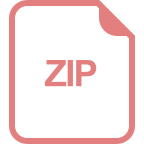
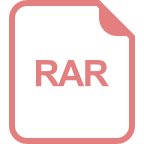
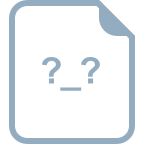
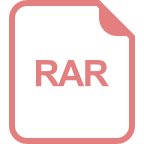