Android如何实现对字符串进行加解密
时间: 2023-12-26 20:03:49 浏览: 298
Android可以通过使用Java中的加解密算法来实现对字符串进行加解密。常见的加解密算法包括AES、DES、RSA等。
以下是一个使用AES加解密字符串的示例代码:
```java
import javax.crypto.Cipher;
import javax.crypto.spec.SecretKeySpec;
import android.util.Base64;
public class AESEncryption {
private static final String ALGORITHM = "AES";
private static final String KEY = "your_key_here"; // 16位或32位的密钥
public static String encrypt(String value) throws Exception {
SecretKeySpec key = generateKey();
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.ENCRYPT_MODE, key);
byte[] encryptedByteValue = cipher.doFinal(value.getBytes("utf-8"));
String encryptedValue64 = Base64.encodeToString(encryptedByteValue, Base64.DEFAULT);
return encryptedValue64;
}
public static String decrypt(String value) throws Exception {
SecretKeySpec key = generateKey();
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.DECRYPT_MODE, key);
byte[] decryptedValue64 = Base64.decode(value, Base64.DEFAULT);
byte[] decryptedByteValue = cipher.doFinal(decryptedValue64);
String decryptedValue = new String(decryptedByteValue,"utf-8");
return decryptedValue;
}
private static SecretKeySpec generateKey() throws Exception {
byte[] keyAsBytes;
keyAsBytes = KEY.getBytes("utf-8");
SecretKeySpec keySpec = new SecretKeySpec(keyAsBytes, ALGORITHM);
return keySpec;
}
}
```
在使用时,可以使用`encrypt()`方法对需要加密的字符串进行加密,使用`decrypt()`方法对加密后的字符串进行解密。注意,加解密时需要使用相同的密钥。
阅读全文
相关推荐
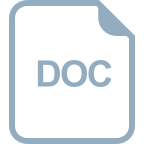
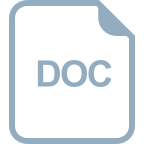
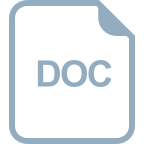
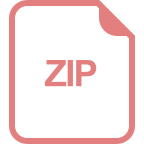
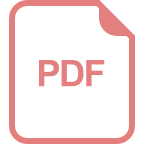
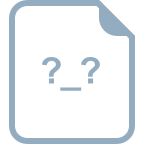
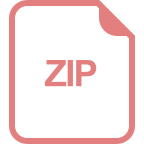



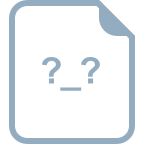
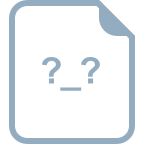
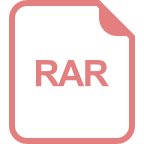
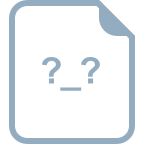
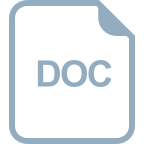
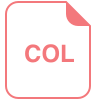
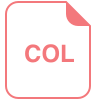

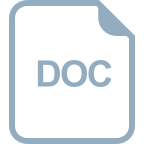