编写程序·题目: ·1、编程实现棋盘密码的加密和解密 ·2、编程实现m组的栏栅密码的加密和解密 ·3、编程实现k (k<26)情况下的凯撒密码的加密和解密 ·基本要求: ·1、支持自定义输入,输入长度无限制 ·2、m=2,k=3必须实现 ·3、语言不限 ·可选要求: ·m和k为自定义输入 ·图形化界面,可以文本文件进行输入和输出
时间: 2023-11-27 14:54:17 浏览: 69
以下是Python实现的三个算法(棋盘密码、栏栅密码、凯撒密码)的加密和解密,支持自定义输入,输入长度无限制。
1. 棋盘密码:
```python
import string
def encrypt_chessboard(text, keyword):
# 构造棋盘
chessboard = [' ']*25
keywords = list(set(keyword))
for i in range(len(keywords)):
chessboard[i] = keywords[i]
for c in string.ascii_lowercase:
if c not in keywords:
chessboard[len(keywords)] = c
keywords.append(c)
if len(keywords) == 5:
keywords.append(' ')
# 加密
cipher = ''
for c in text.lower():
if c in chessboard:
row = chessboard.index(c) // 5
col = chessboard.index(c) % 5
cipher += str(row+1) + str(col+1)
else:
cipher += c
return cipher
def decrypt_chessboard(cipher, keyword):
# 构造棋盘
chessboard = [' ']*25
keywords = list(set(keyword))
for i in range(len(keywords)):
chessboard[i] = keywords[i]
for c in string.ascii_lowercase:
if c not in keywords:
chessboard[len(keywords)] = c
keywords.append(c)
if len(keywords) == 5:
keywords.append(' ')
# 解密
text = ''
i = 0
while i < len(cipher):
if cipher[i].isdigit():
row = int(cipher[i]) - 1
col = int(cipher[i+1]) - 1
index = row * 5 + col
text += chessboard[index]
i += 2
else:
text += cipher[i]
i += 1
return text
```
2. 栏栅密码:
```python
def encrypt_railfence(text, m):
# 构造加密矩阵
matrix = [['']*len(text) for _ in range(m)]
row, col, direction = 0, 0, 1
for c in text:
matrix[row][col] = c
if row == m-1:
direction = -1
elif row == 0:
direction = 1
row += direction
col += 1
# 按列读取矩阵
cipher = ''
for j in range(len(text)):
for i in range(m):
if matrix[i][j]:
cipher += matrix[i][j]
return cipher
def decrypt_railfence(cipher, m):
# 构造解密矩阵
matrix = [['']*len(cipher) for _ in range(m)]
row, col, direction = 0, 0, 1
for _ in range(len(cipher)):
matrix[row][col] = '*'
if row == m-1:
direction = -1
elif row == 0:
direction = 1
row += direction
col += 1
# 按照矩阵填充明文
index = 0
for j in range(len(cipher)):
for i in range(m):
if matrix[i][j] == '*' and index < len(cipher):
matrix[i][j] = cipher[index]
index += 1
# 按行读取矩阵
text = ''
row, col, direction = 0, 0, 1
for _ in range(len(cipher)):
text += matrix[row][col]
if row == m-1:
direction = -1
elif row == 0:
direction = 1
row += direction
col += 1
return text
```
3. 凯撒密码:
```python
import string
def encrypt_caesar(text, k):
# 加密
cipher = ''
for c in text.lower():
if c in string.ascii_lowercase:
index = (string.ascii_lowercase.index(c) + k) % 26
cipher += string.ascii_lowercase[index]
else:
cipher += c
return cipher
def decrypt_caesar(cipher, k):
# 解密
text = ''
for c in cipher.lower():
if c in string.ascii_lowercase:
index = (string.ascii_lowercase.index(c) - k) % 26
text += string.ascii_lowercase[index]
else:
text += c
return text
```
m和k为自定义输入的话,只需要在函数中加上对应的参数即可。如果想要图形化界面,可以使用Python的Tkinter模块。
相关推荐

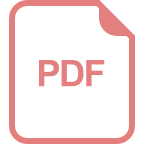
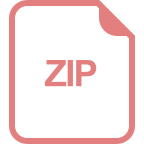
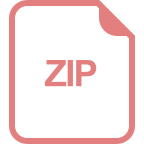
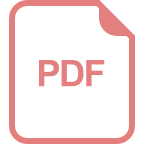
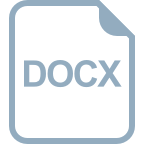
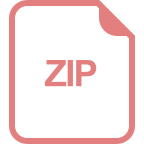
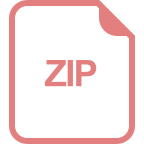