制作一个安卓简单的app
时间: 2023-10-11 13:07:25 浏览: 64
好的,制作一个安卓简单的app需要以下步骤:
1. 确定app的功能和需求,例如是一个计算器app或者一个天气预报app等等。
2. 下载并安装Android Studio,这是一个官方提供的开发工具,可以帮助你快速制作出安卓app。
3. 在Android Studio中创建一个新的工程,并设置好app的基本信息,如包名、应用名称、图标等。
4. 设计app的用户界面,使用Android Studio提供的布局编辑器来创建UI界面,添加各种控件和布局,如文本框、按钮、列表等。
5. 编写app的业务逻辑和功能代码,使用Java或Kotlin语言编写代码,实现各种功能,如数据处理、网络请求、界面交互等。
6. 调试和测试app,确保app的各项功能都能正常运行,并且没有任何bug或者crash。
7. 发布app,将app发布到Google Play商店或者其他应用商店,让更多人使用你的app。
以上是制作一个安卓简单的app的基本步骤,当然具体的实现还需要根据你的具体需求进行调整。希望对你有所帮助。
相关问题
Android studio 制作一个点外卖app
好的,下面是Android Studio制作一个点外卖App的简要步骤:
1. 首先,您需要创建一个新的Android Studio项目。在创建项目时,您可以选择空模板或其他适合您的模板。
2. 接下来,您需要设计和创建应用程序UI界面。您可以使用Android Studio自带的可视化布局编辑器,或者手动编写XML布局文件来实现。
3. 在UI设计完成后,您需要添加代码来实现应用程序的功能。例如,您需要编写代码来获取用户的位置信息、搜索附近的餐厅、浏览菜单、下单、支付等。
4. 在代码编写完成后,您需要对应用程序进行调试和测试。您可以使用Android Studio自带的模拟器或者将应用程序安装到真实的设备上进行测试。
5. 最后,您需要将应用程序打包并发布到应用商店。在发布应用程序之前,您需要确保应用程序符合相关的法律法规和应用商店的规定。
以上是一个简单的制作点外卖App的步骤,当然具体的实现过程需要根据您的具体需求和技能水平而定。希望这对您有所帮助!
android制作一个简单j的记事本app
要制作一个简单的记事本应用,你需要做以下几步:
1. 创建一个新的Android项目,包含一个空白的Activity;
2. 在布局文件中添加一个EditText控件用于输入文本,一个Button控件用于保存文本;
3. 在Activity中获取EditText和Button控件,并为Button添加一个点击事件;
4. 在点击事件中获取EditText中的文本内容,并将其保存到SharedPreferences中;
5. 在Activity的onCreate方法中,从SharedPreferences中读取之前保存的文本内容,并将其显示在EditText中。
下面是示例代码:
1. 在布局文件中添加EditText和Button控件:
```xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<EditText
android:id="@+id/edit_text"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="请输入文本内容"/>
<Button
android:id="@+id/save_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="保存"/>
</LinearLayout>
```
2. 在Activity中获取EditText和Button控件,并为Button添加一个点击事件:
```java
public class MainActivity extends AppCompatActivity {
private EditText mEditText;
private Button mSaveButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mEditText = findViewById(R.id.edit_text);
mSaveButton = findViewById(R.id.save_button);
mSaveButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
saveText();
}
});
}
private void saveText() {
String text = mEditText.getText().toString();
SharedPreferences preferences = getSharedPreferences("MyNotes", MODE_PRIVATE);
SharedPreferences.Editor editor = preferences.edit();
editor.putString("Note", text);
editor.apply();
Toast.makeText(this, "保存成功!", Toast.LENGTH_SHORT).show();
}
}
```
3. 在点击事件中获取EditText中的文本内容,并将其保存到SharedPreferences中。
4. 在Activity的onCreate方法中,从SharedPreferences中读取之前保存的文本内容,并将其显示在EditText中:
```java
public class MainActivity extends AppCompatActivity {
private EditText mEditText;
private Button mSaveButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mEditText = findViewById(R.id.edit_text);
mSaveButton = findViewById(R.id.save_button);
mSaveButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
saveText();
}
});
SharedPreferences preferences = getSharedPreferences("MyNotes", MODE_PRIVATE);
String note = preferences.getString("Note", "");
mEditText.setText(note);
}
private void saveText() {
String text = mEditText.getText().toString();
SharedPreferences preferences = getSharedPreferences("MyNotes", MODE_PRIVATE);
SharedPreferences.Editor editor = preferences.edit();
editor.putString("Note", text);
editor.apply();
Toast.makeText(this, "保存成功!", Toast.LENGTH_SHORT).show();
}
}
```
这样,你就可以创建一个简单的记事本应用了。当用户输入文本并点击保存按钮时,文本内容将被保存到SharedPreferences中,并在下次打开应用时自动显示在EditText中。
相关推荐
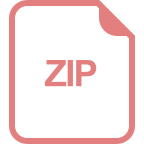
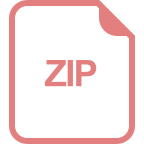












