vue3+ts按钮互斥
时间: 2023-07-01 10:12:42 浏览: 75
如果你想实现在多个按钮中只能选择一个按钮的互斥效果,可以考虑以下两种方式:
1. 使用单选框(Radio)组件
使用 Vue 3 的单选框组件可以轻松实现多个按钮中只能选择一个的互斥效果。你可以在模板中使用 `v-model` 指令绑定一个选中的值,然后将多个单选框的值绑定到同一个数据属性上,这样就可以实现互斥效果。
```html
<template>
<div>
<label>
<input type="radio" v-model="selected" value="option1" /> Option 1
</label>
<label>
<input type="radio" v-model="selected" value="option2" /> Option 2
</label>
<label>
<input type="radio" v-model="selected" value="option3" /> Option 3
</label>
</div>
</template>
<script>
import { defineComponent, ref } from 'vue'
export default defineComponent({
setup() {
const selected = ref('option1')
return {
selected,
}
},
})
</script>
```
2. 使用按钮组件并在点击事件中切换状态
如果你想使用按钮组件来实现互斥效果,可以在模板中使用 `v-for` 循环渲染多个按钮,并在点击事件中切换按钮的激活状态。你可以使用一个 `active` 数据属性来记录当前选中的按钮,并在点击事件中将其切换为对应的按钮。
```html
<template>
<div>
<button
v-for="option in options"
:key="option.value"
:class="{ active: active === option.value }"
@click="handleClick(option.value)"
>
{{ option.label }}
</button>
</div>
</template>
<script>
import { defineComponent, ref } from 'vue'
export default defineComponent({
setup() {
const options = [
{ label: 'Option 1', value: 'option1' },
{ label: 'Option 2', value: 'option2' },
{ label: 'Option 3', value: 'option3' },
]
const active = ref('option1')
const handleClick = (value) => {
active.value = value
}
return {
options,
active,
handleClick,
}
},
})
</script>
<style>
.active {
background-color: blue;
color: white;
}
</style>
```
希望这些内容能够帮到你!
相关推荐
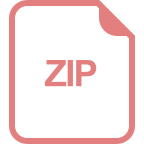
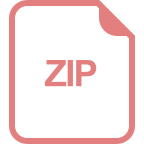














