Consider two 4-bit binary numbers A and B. Bits of A are a3, a2, a1, and a0, and bits of B are b3, b2, b1, and b0. A is greater than B if a3 is 1 and b3 is 0, but if a3 and b3 are the same, then if a2 is 1 and b2 is 0, we can determine that A is greater than B. This evaluation continues until a0 and b0 are considered. If a0 and b0 are equal then A and B are equal. Using discrete gates and Verilog gate primitives build a 4-bit comparator that generates a 1 on its GT output when its 4-bit input A is greater than its B input, and a 1 on its EQ output when A and B are equal. You must use the generate statement and other Verilog iterative logic support structures.
时间: 2023-12-24 18:02:56 浏览: 59
Here is the Verilog code for a 4-bit comparator that generates a 1 on its GT output when input A is greater than B, and a 1 on its EQ output when A equals B:
```
module comparator (
input [3:0] A,
input [3:0] B,
output reg GT,
output reg EQ
);
generate
genvar i;
for (i = 3; i >= 0; i--) begin
if (i == 3) begin
always @(*) begin
if (A[3] == 1 && B[3] == 0) begin
GT = 1;
EQ = 0;
end
else if (A[3] == B[3]) begin
GT = 0;
EQ = 1;
end
else begin
GT = 0;
EQ = 0;
end
end
end
else begin
always @(*) begin
if (GT == 1 || (A[i] == 1 && B[i] == 0)) begin
GT = 1;
EQ = 0;
end
else if (EQ == 1 || A[i] == B[i]) begin
GT = 0;
EQ = 1;
end
else begin
GT = 0;
EQ = 0;
end
end
end
end
endgenerate
endmodule
```
In this code, we use a generate statement to create a loop that iterates over each bit of the input. For each bit, we use an always block with a sensitivity list of all inputs and outputs to determine the values of GT and EQ. At each bit position, we compare the corresponding bits of A and B, and set GT or EQ according to the rules given in the problem statement. We also use the values of GT and EQ from previous bit positions to determine the values for the current bit.
阅读全文
相关推荐
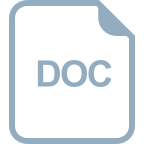
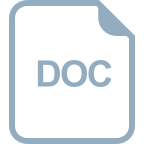
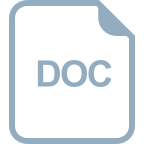

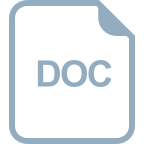
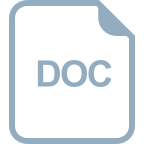
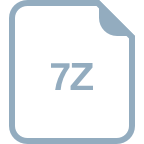
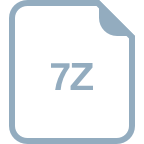
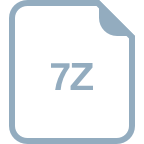
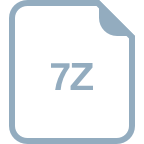
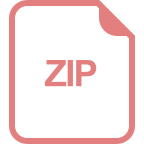
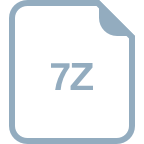
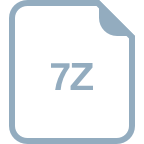
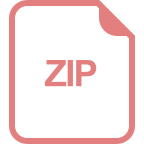
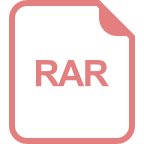