give me a complete verilog code according this file(base on basy3)
时间: 2024-12-16 09:18:36 浏览: 6
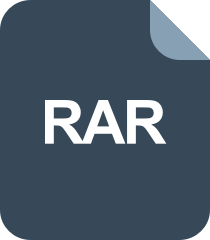
c_a.rar_C/A码_GPS VHDL_GPS Verilog code_GPS verilog_c_a
Certainly! Below is a complete Verilog code for implementing the VGA interface and the wrapper module based on the BASYS 3 board. This code includes the necessary components to control the VGA display and test the functionality.
### VGA Interface Module (`vga_interface.v`)
```verilog
module vga_interface (
input wire CLK,
input wire [11:0] COLOUR_IN,
output reg [11:0] COLOUR_OUT,
output reg HS,
output reg VS,
output reg [9:0] X,
output reg [9:0] Y
);
// Parameters for timing
parameter H_FRONT_PORCH = 16;
parameter H_SYNC_WIDTH = 96;
parameter H_BACK_PORCH = 48;
parameter H_DISPLAY = 640;
parameter V_FRONT_PORCH = 10;
parameter V_SYNC_WIDTH = 2;
parameter V_BACK_PORCH = 33;
parameter V_DISPLAY = 480;
// Internal counters
reg [9:0] h_count;
reg [9:0] v_count;
// Synchronization signals
always @(posedge CLK) begin
if (h_count == 799) begin
h_count <= 0;
if (v_count == 520) begin
v_count <= 0;
end else begin
v_count <= v_count + 1;
end
end else begin
h_count <= h_count + 1;
end
end
// Horizontal Sync
assign HS = (h_count >= H_FRONT_PORCH && h_count < (H_FRONT_PORCH + H_SYNC_WIDTH)) ? 1'b0 : 1'b1;
// Vertical Sync
assign VS = (v_count >= V_FRONT_PORCH && v_count < (V_FRONT_PORCH + V_SYNC_WIDTH)) ? 1'b0 : 1'b1;
// Pixel coordinates
assign X = (h_count < H_FRONT_PORCH + H_SYNC_WIDTH + H_BACK_PORCH) ? 10'd0 : (h_count - (H_FRONT_PORCH + H_SYNC_WIDTH + H_BACK_PORCH));
assign Y = (v_count < V_FRONT_PORCH + V_SYNC_WIDTH + V_BACK_PORCH) ? 10'd0 : (v_count - (V_FRONT_PORCH + V_SYNC_WIDTH + V_BACK_PORCH));
// Valid signal
wire valid = (X < H_DISPLAY) && (Y < V_DISPLAY);
// Color output
always @(posedge CLK) begin
if (valid) begin
COLOUR_OUT <= COLOUR_IN;
end else begin
COLOUR_OUT <= 12'd0;
end
end
endmodule
```
### Wrapper Module (`vga_wrapper.v`)
```verilog
module vga_wrapper (
input wire CLK,
input wire [11:0] SW, // Slide switches
output wire [11:0] COLOUR_OUT,
output wire HS,
output wire VS,
output wire [9:0] X,
output wire [9:0] Y
);
// Internal signals
reg [11:0] colour_in;
// Instantiate the VGA interface
vga_interface vga_inst (
.CLK(CLK),
.COLOUR_IN(colour_in),
.COLOUR_OUT(COLOUR_OUT),
.HS(HS),
.VS(VS),
.X(X),
.Y(Y)
);
// Determine the color based on the position
always @(*) begin
colour_in = SW; // Default color from slide switches
if ((X >= 0 && X < 10) && (Y >= 0 && Y < 10)) begin
colour_in = ~SW; // Invert color in top-left corner
end else if ((X >= 630 && X < 640) && (Y >= 0 && Y < 10)) begin
colour_in = ~SW; // Invert color in top-right corner
end else if ((X >= 0 && X < 10) && (Y >= 470 && Y < 480)) begin
colour_in = ~SW; // Invert color in bottom-left corner
end else if ((X >= 630 && X < 640) && (Y >= 470 && Y < 480)) begin
colour_in = ~SW; // Invert color in bottom-right corner
end
end
endmodule
```
### Constraints File (`vga.xdc`)
```tcl
# VGA pins
set_property PACKAGE_PIN E3 [get_ports {CLK}]
set_property IOSTANDARD LVCMOS33 [get_ports {CLK}]
set_property PACKAGE_PIN G19 [get_ports {HS}]
set_property IOSTANDARD LVCMOS33 [get_ports {HS}]
set_property PACKAGE_PIN J19 [get_ports {VS}]
set_property IOSTANDARD LVCMOS33 [get_ports {VS}]
set_property PACKAGE_PIN R18 [get_ports {X[0]}]
set_property PACKAGE_PIN T18 [get_ports {X[1]}]
set_property PACKAGE_PIN U18 [get_ports {X[2]}]
set_property PACKAGE_PIN N18 [get_ports {X[3]}]
set_property PACKAGE_PIN P18 [get_ports {X[4]}]
set_property PACKAGE_PIN K18 [get_ports {X[5]}]
set_property PACKAGE_PIN M18 [get_ports {X[6]}]
set_property PACKAGE_PIN L18 [get_ports {X[7]}]
set_property PACKAGE_PIN J18 [get_ports {X[8]}]
set_property PACKAGE_PIN H18 [get_ports {X[9]}]
set_property PACKAGE_PIN R17 [get_ports {Y[0]}]
set_property PACKAGE_PIN T17 [get_ports {Y[1]}]
set_property PACKAGE_PIN U17 [get_ports {Y[2]}]
set_property PACKAGE_PIN N17 [get_ports {Y[3]}]
set_property PACKAGE_PIN P17 [get_ports {Y[4]}]
set_property PACKAGE_PIN K17 [get_ports {Y[5]}]
set_property PACKAGE_PIN M17 [get_ports {Y[6]}]
set_property PACKAGE_PIN L17 [get_ports {Y[7]}]
set_property PACKAGE_PIN J17 [get_ports {Y[8]}]
set_property PACKAGE_PIN H17 [get_ports {Y[9]}]
set_property PACKAGE_PIN T16 [get_ports {COLOUR_OUT[0]}]
set_property PACKAGE_PIN U16 [get_ports {COLOUR_OUT[1]}]
set_property PACKAGE_PIN R16 [get_ports {COLOUR_OUT[2]}]
set_property PACKAGE_PIN T15 [get_ports {COLOUR_OUT[3]}]
set_property PACKAGE_PIN U15 [get_ports {COLOUR_OUT[4]}]
set_property PACKAGE_PIN R15 [get_ports {COLOUR_OUT[5]}]
set_property PACKAGE_PIN T14 [get_ports {COLOUR_OUT[6]}]
set_property PACKAGE_PIN U14 [get_ports {COLOUR_OUT[7]}]
set_property PACKAGE_PIN R14 [get_ports {COLOUR_OUT[8]}]
set_property PACKAGE_PIN T13 [get_ports {COLOUR_OUT[9]}]
set_property PACKAGE_PIN U13 [get_ports {COLOUR_OUT[10]}]
set_property PACKAGE_PIN R13 [get_ports {COLOUR_OUT[11]}]
# Slide switches
set_property PACKAGE_PIN W12 [get_ports {SW[0]}]
set_property PACKAGE_PIN V12 [get_ports {SW[1]}]
set_property PACKAGE_PIN T12 [get_ports {SW[2]}]
set_property PACKAGE_PIN R12 [get_ports {SW[3]}]
set_property PACKAGE_PIN W11 [get_ports {SW[4]}]
set_property PACKAGE_PIN V11 [get_ports {SW[5]}]
set_property PACKAGE_PIN T11 [get_ports {SW[6]}]
set_property PACKAGE_PIN R11 [get_ports {SW[7]}]
set_property PACKAGE_PIN W10 [get_ports {SW[8]}]
set_property PACKAGE_PIN V10 [get_ports {SW[9]}]
set_property PACKAGE_PIN T10 [get_ports {SW[10]}]
set_property PACKAGE_PIN R10 [get_ports {SW[11]}]
# Clock constraint
create_clock -name sys_clk -period 10.000 -waveform {0 5} [get_ports CLK]
```
### Steps to Implement and Test
1. **Create Project**: Open Vivado and create a new project.
2. **Add Source Files**: Add `vga_interface.v`, `vga_wrapper.v`, and `vga.xdc` to your project.
3. **Set Top Module**: Set `vga_wrapper` as the top module.
4. **Run Synthesis and Implementation**: Run synthesis and implementation.
5. **Generate Bitstream**: Generate the bitstream file.
6. **Program FPGA**: Program the FPGA using the generated `.bit` file.
7. **Connect Monitor**: Connect the BASYS 3 board to your monitor using the VGA cable and select the appropriate input source on the monitor.
8. **Test**: Change the configuration of the slide switches to see how they affect the colors on the display.
Feel free to modify the code to add additional features or improve performance as needed.
阅读全文
相关推荐
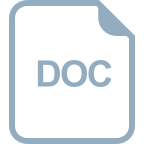
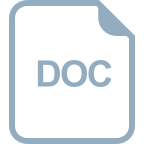
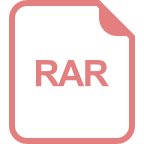
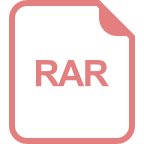
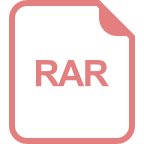
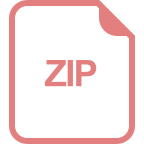
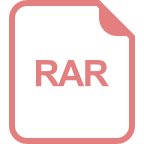
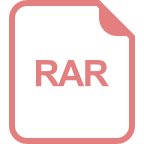
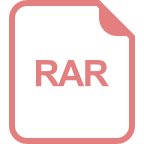
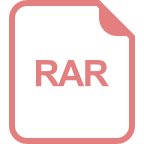
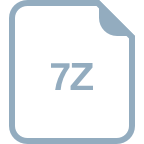
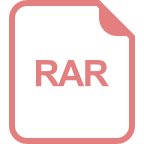
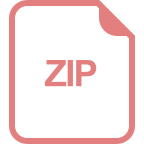
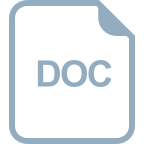
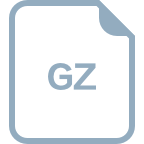