C语言程序。输入一行以句号(.)结尾的字符串。首先输出将其中大、小写字母颠倒后得到的新句子,接下来依次输出该字符串中字母、空格、数字和其他字符(标点符号等)的数目。
时间: 2024-11-18 19:27:57 浏览: 30
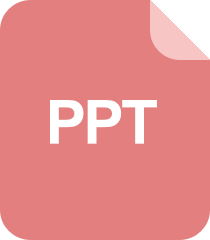
字符串的输入输出-语言课程课件
C语言程序通常会包括预处理指令、函数声明和主函数。下面是一个简单的示例程序,用于实现你所描述的功能:
```c
#include <stdio.h>
#include <ctype.h>
void reverse_case(char *str);
int count_chars(char *str);
int main() {
char input[100];
printf("请输入一行以句号(.)结尾的字符串: ");
fgets(input, sizeof(input), stdin); // 读取用户输入,忽略行结束符
input[strcspn(input, "\n")] = '\0'; // 去除换行符
reverse_case(input);
printf("颠倒大小写后的字符串: %s\n", input);
int alpha_count = count_chars(input);
int space_count = strcspn(input, " ");
int digit_count = count_digits(input);
int other_count = strlen(input) - (alpha_count + space_count + digit_count);
printf("字母数: %d, 空格数: %d, 数字数: %d, 其他字符数: %d\n", alpha_count, space_count, digit_count, other_count);
return 0;
}
// 函数:反转字符串中的大、小写字母
void reverse_case(char *str) {
for (int i = 0; i < strlen(str); ++i) {
if (islower(str[i])) {
str[i] = toupper(str[i]);
} else if (isupper(str[i])) {
str[i] = tolower(str[i]);
}
}
}
// 函数:计算字符数量(仅统计字母、空格、数字)
int count_chars(char *str) {
int count = 0;
for (char c : str) {
if (isalnum(c)) { // 检查是否为字母或数字
count++;
}
}
return count;
}
```
当你运行这个程序,它会让你输入一行字符串,并按照要求显示结果。
阅读全文
相关推荐
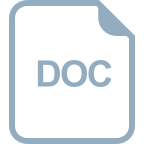
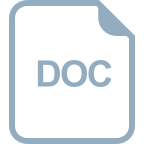
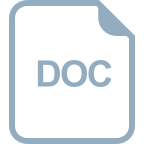
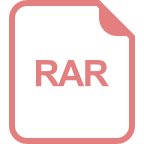
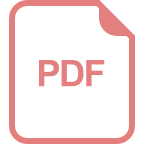
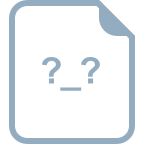
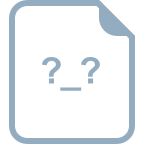
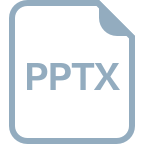
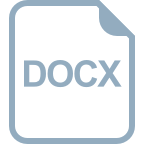
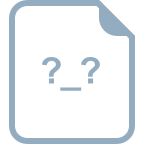
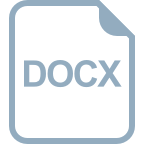
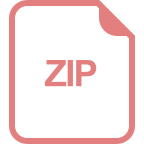