用C语言写一个ELF文件的解析器
时间: 2023-12-04 21:05:08 浏览: 136
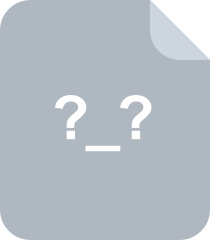
解析ELF文件

当然可以!以下是一个简单的 ELF 文件解析器的 C 语言代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <elf.h>
int main(int argc, char **argv) {
if (argc != 2) {
fprintf(stderr, "Usage: %s <ELF file>\n", argv[0]);
exit(EXIT_FAILURE);
}
FILE *file = fopen(argv[1], "rb");
if (file == NULL) {
perror("Failed to open file");
exit(EXIT_FAILURE);
}
Elf64_Ehdr header;
if (fread(&header, sizeof(header), 1, file) != 1) {
perror("Failed to read ELF header");
exit(EXIT_FAILURE);
}
printf("ELF header:\n");
printf(" Magic number: %02x %02x %02x %02x\n", header.e_ident[EI_MAG0], header.e_ident[EI_MAG1], header.e_ident[EI_MAG2], header.e_ident[EI_MAG3]);
printf(" Class: %u-bit\n", header.e_ident[EI_CLASS] == ELFCLASS32 ? 32 : 64);
printf(" Data encoding: %s-endian\n", header.e_ident[EI_DATA] == ELFDATA2LSB ? "little" : "big");
printf(" Version: %u\n", header.e_ident[EI_VERSION]);
printf(" OS ABI: %u\n", header.e_ident[EI_OSABI]);
printf(" ABI version: %u\n", header.e_ident[EI_ABIVERSION]);
printf(" Type: %u\n", header.e_type);
printf(" Machine: %u\n", header.e_machine);
printf(" Version: %u\n", header.e_version);
printf(" Entry point address: 0x%lx\n", header.e_entry);
printf(" Program header offset: %lu\n", header.e_phoff);
printf(" Section header offset: %lu\n", header.e_shoff);
printf(" Flags: %u\n", header.e_flags);
printf(" ELF header size: %u\n", header.e_ehsize);
printf(" Program header entry size: %u\n", header.e_phentsize);
printf(" Number of program header entries: %u\n", header.e_phnum);
printf(" Section header entry size: %u\n", header.e_shentsize);
printf(" Number of section header entries: %u\n", header.e_shnum);
printf(" Section name string table index: %u\n", header.e_shstrndx);
if (fseek(file, header.e_shoff, SEEK_SET) != 0) {
perror("Failed to seek to section header table");
exit(EXIT_FAILURE);
}
Elf64_Shdr section_header;
for (int i = 0; i < header.e_shnum; i++) {
if (fread(§ion_header, sizeof(section_header), 1, file) != 1) {
perror("Failed to read section header");
exit(EXIT_FAILURE);
}
printf("Section %d:\n", i);
printf(" Name: %u\n", section_header.sh_name);
printf(" Type: %lu\n", section_header.sh_type);
printf(" Flags: %lu\n", section_header.sh_flags);
printf(" Address: 0x%lx\n", section_header.sh_addr);
printf(" Offset: %lu\n", section_header.sh_offset);
printf(" Size: %lu\n", section_header.sh_size);
printf(" Link: %u\n", section_header.sh_link);
printf(" Info: %u\n", section_header.sh_info);
printf(" Address alignment: %lu\n", section_header.sh_addralign);
printf(" Entry size: %lu\n", section_header.sh_entsize);
}
fclose(file);
return EXIT_SUCCESS;
}
```
这个解析器使用标准 C 库函数和 ELF 头文件,打开指定的 ELF 文件,读取和解析 ELF 文件头部信息和节头表信息,并将它们打印到标准输出中。注意这个解析器仅适用于 64 位 ELF 文件,并且没有处理节的内容。
阅读全文
相关推荐
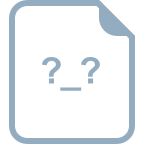
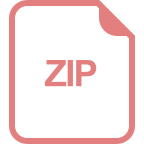
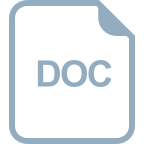
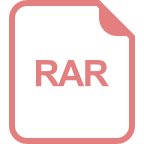
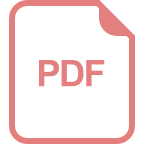
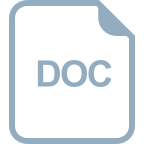
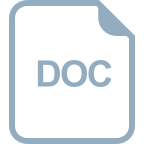
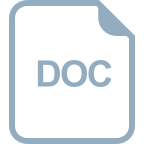
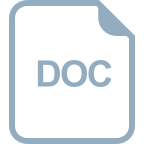
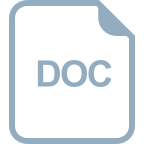
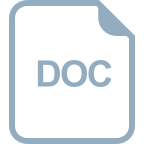
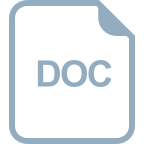
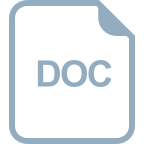
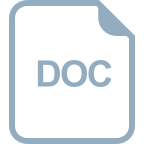
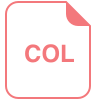
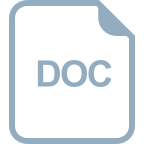
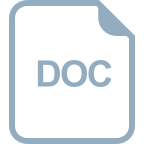