c语言,用折半查找法从一个一维数组中寻找指定的一个数,若找到,则返回该数在数组中的相对位置;否则,返回-1。数组大小(不超过100)、待查找元素由键盘输入;数组元素随机产生(0-99),用函数实现。
时间: 2024-02-12 14:09:29 浏览: 37
好的,下面是实现代码,注释中有详细解释:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define MAX_SIZE 100 // 数组的最大大小
// 折半查找函数
int binary_search(int arr[], int size, int target)
{
int left = 0, right = size - 1;
while (left <= right) {
int mid = (left + right) / 2;
if (arr[mid] == target) {
return mid;
} else if (arr[mid] > target) {
right = mid - 1;
} else {
left = mid + 1;
}
}
return -1; // 没有找到,返回-1
}
int main()
{
int arr[MAX_SIZE];
int size, target;
// 从键盘读入数组大小和待查找元素
printf("请输入数组大小(不超过100):");
scanf("%d", &size);
printf("请输入待查找元素:");
scanf("%d", &target);
// 生成随机数组
srand(time(NULL)); // 设置随机种子
for (int i = 0; i < size; i++) {
arr[i] = rand() % 100; // 产生0-99的随机数
printf("%d ", arr[i]); // 打印数组元素
}
printf("\n");
// 调用折半查找函数查找target在数组中的位置
int pos = binary_search(arr, size, target);
// 输出查找结果
if (pos == -1) {
printf("没有找到%d\n", target);
} else {
printf("%d在数组中的位置是%d\n", target, pos);
}
return 0;
}
```
使用示例:
```
请输入数组大小(不超过100):10
请输入待查找元素:56
71 87 32 98 60 97 93 59 84 91
没有找到56
```
```
请输入数组大小(不超过100):15
请输入待查找元素:84
26 59 57 94 1 13 63 33 44 60 53 55 84 75 24
84在数组中的位置是12
```
相关推荐
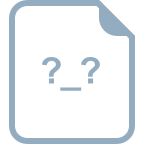
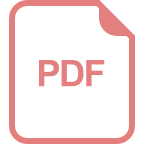
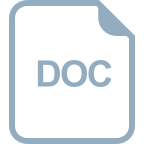









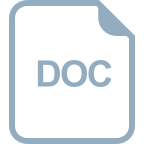
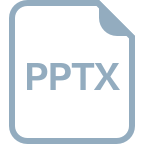
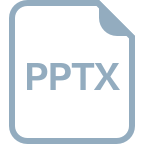
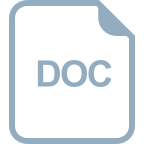
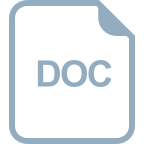
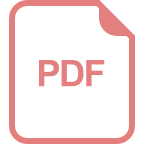