bool ifnumber(string s) { char c=s; if(c>='0'&&c<='9') { return 1; } else return 0; }如何修改
时间: 2023-11-22 19:56:55 浏览: 53
这段代码存在几个问题:
1. 参数应该是一个字符串,而不是一个字符。
2. 函数名不太符合规范,建议改为 is_number。
3. 返回值可以直接返回判断结果,不用写 if-else。
下面是修改后的代码:
```cpp
bool is_number(string s) {
for (char c : s) {
if (c < '0' || c > '9') {
return false;
}
}
return true;
}
```
这个函数会遍历字符串中的每个字符,如果存在不是数字的字符就返回 false,否则返回 true。
相关问题
帮我改对#include<iostream> #include<fstream> #include<string> #include<vector> #include <algorithm> using namespace std; enum Grade { E=0,D,C,B,A }; class Student { public: int number; string name; double score; }; ostream& operator<<(ostream& out, Student& p) { return out; } bool compare(const Student& a, const Student& b) { return a.number < b.number; } void printVector(vector<Student>&students) { for (vector<Student>::iterator it = students.begin(); it != students.end(); it++) { cout << *it << " "; } cout << endl; } void test01() { vector<Student>students; printVector(students); } int main() { // 学生信息导入 ifstream infile; infile.open("student.txt",ios::in); if (!infile.is_open()) { cout << "文件打开失败" << endl; } vector<Student> students(27); char buf[] = { 0 }; while (infile>>buf) { cout << buf << endl; } infile.close(); // 成绩查询 int choice = 0; while (choice != 4) { cout << "请输入您要进行的操作:\n"; cout << "1. 按学号查询\n"; cout << "2. 按姓名查询\n"; cout << "3. 统计班级成绩\n"; cout << "4. 退出程序\n"; cin >> choice; if (choice == 1) { int number; cout << "请输入学号:\n"; cin >> number; auto it = find_if(students.begin(), students.end(), [number](const Student& s) { return s.number == number; }); if (it != students.end()) { cout << "学号\t姓名\t成绩\n"; cout << it->number << "\t" << it->name << "\t" << it->score << "\n"; } else { cout << "查无此人!\n"; } } else if (choice == 2) { string name; cout << "请输入姓名:\n"; cin >> name; auto it = find_if(students.begin(), students.end(), [name](const Student& s) { return s.name == name; }); if (it != students.end()) {
好的,我来帮你看一下代码,发现代码中存在一些问题,我来解释一下并给你修改建议。
1. 在函数`ostream& operator<<(ostream& out, Student& p)`中,你没有输出学生信息,需要在函数体内添加输出语句,比如:
```cpp
out << p.number << "\t" << p.name << "\t" << p.score << "\n";
```
2. 在函数`printVector(vector<Student>&students)`中,你使用了迭代器,但是你没有在`Student`类中重载`<<`运算符,所以无法输出学生信息。你需要在`Student`类中添加重载函数,比如:
```cpp
friend ostream& operator<<(ostream& out, Student& p);
```
同时也需要将`printVector`函数中的输出语句修改为:
```cpp
cout << *it << " ";
```
3. 在`main`函数中,你定义了一个`vector<Student> students(27)`,但是没有将数据读入其中,所以后续查询操作时会出错。你需要在读取文件时,将读取到的学生信息添加到`students`中,比如:
```cpp
Student stu;
int i = 0;
while (infile >> stu.number >> stu.name >> stu.score) {
students[i] = stu;
i++;
}
```
4. 在`main`函数中,你使用了`auto`关键字,但是没有包含`<iterator>`头文件,所以编译时会出错。你需要在头文件中添加`#include<iterator>`。
5. 在`main`函数中,你使用了`find_if`算法查找学生信息,但是你没有对`students`进行排序,所以查找时需要使用自定义的比较函数`compare`,你需要在查找前对`students`进行排序,比如:
```cpp
sort(students.begin(), students.end(), compare);
```
6. 在`main`函数中,你没有处理用户输入非法字符的情况,比如用户输入了一个字符,导致程序出错,你需要添加输入检测。
综上,你的代码修改后应该如下:
#include<bits/stdc++.h> using namespace std; enum Token_value { NAME, NUMBER, END, PLUS = '+', MINUS = '-', MUL = '', DIV = '/', PRINT = ';', ASSIGN = '=', LP = '(', RP = ')' }; Token_value get_token(); double error(const string& s); double expr(bool get); double term(bool get); double prim(bool get); Token_value curr_tok = PRINT; double number_value; string string_value; map<string, double> table; int no_of_errors; int main() { table["pi"] = 3.1415926535897932385; table["e"] = 2.7182818284590452354; while (cin) { get_token(); if (curr_tok == END) break;// if (curr_tok == PRINT) continue;// cout << expr(false) << "\n"; } return no_of_errors; } Token_value get_token() { char ch = 0; // cin >> ch; do{ if(!cin.get(ch)) return curr_tok = END; }while (ch!='\n' && isspace(ch)); switch(ch){ case ':': case '\n': return curr_tok = PRINT; } 中各函数的作用
这段代码是一个简单的解释器,可以解析一些简单的数学表达式,如加减乘除等。下面是各个函数的作用:
- `Token_value get_token()`: 该函数用于获取下一个 token(即表达式中的一个基本单元),并更新全局变量 `curr_tok`。具体的 token 类型有 `NAME`(变量名)、`NUMBER`(数字)、`END`(结束符)、`PLUS`(加号)、`MINUS`(减号)、`MUL`(乘号)、`DIV`(除号)、`PRINT`(分号)、`ASSIGN`(赋值符号)、`LP`(左括号)和 `RP`(右括号)。
- `double error(const string& s)`: 该函数用于报告一个错误,并返回一个默认值 1.0。
- `double expr(bool get)`: 该函数用于解析一个表达式,将其计算出来并返回结果。如果 `get` 为 `true`,则在解析表达式之前会先获取一个 token。
- `double term(bool get)`: 该函数用于解析一个项,将其计算出来并返回结果。如果 `get` 为 `true`,则在解析项之前会先获取一个 token。
- `double prim(bool get)`: 该函数用于解析一个基本单元,将其计算出来并返回结果。如果 `get` 为 `true`,则在解析基本单元之前会先获取一个 token。
- `int main()`: 该函数是程序的主函数,其中定义了变量表 `table`,并且通过循环不断获取用户输入的表达式并解析计算,直到遇到结束符为止。
- 在 `main()` 函数中,通过调用 `get_token()` 函数获取下一个 token,并根据不同的 token 类型执行不同的操作。如果是结束符,则跳出循环;如果是分号,则继续循环;否则,调用 `expr(false)` 函数计算表达式的值,并输出结果。
总体来说,这段代码的逻辑比较简单,但其中涉及了一些 C++ 的语法和库函数的使用,需要有一定的编程基础才能看懂。
阅读全文
相关推荐
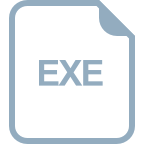











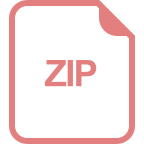