Python编程实现八数码问题
时间: 2024-06-14 22:01:57 浏览: 145
八数码问题,也称为汉诺塔(Tower of Hanoi),是一个经典的递归问题,通常用三个柱子和若干个盘子来表示,目标是将所有盘子从起始柱子A移动到目标柱子C,但在移动过程中,任何时候都不能有大盘子放在小盘子上。这个过程可以用Python编写程序来模拟。
在Python中实现八数码问题,可以使用递归来描述每一步移动。以下是简单的代码示例:
```python
def hanoi(n, source, auxiliary, target):
if n > 0:
# 递归地移动n- 1, source, target, auxiliary)
# 将最大的盘子从源柱子移动到目标柱子
print(f"Move disk {n} from {source} to {target}")
# 最后,如果还有剩余的盘子,将它们从辅助柱子移动到目标柱子
hanoi(n - 1, auxiliary, source, target)
# 调用函数,开始游戏
hanoi(3, 'A', 'B', 'C')
```
当你运行这段代码,它会按照典型的汉诺塔规则一步步地打印出移动的过程。
相关问题
python编程实现回溯搜索法求解该八数码问题,完整代码
在Python中,回溯搜索(Backtracking)是一种解决组合优化问题的有效算法,特别是在解决像8数码(又称数独)这样的逻辑谜题时。以下是使用回溯搜索求解数独的一个基本示例代码:
```python
def is_valid(board, row, col, num):
# 检查行是否合法
for i in range(9):
if board[row][i] == num:
return False
# 检查列是否合法
for i in range(9):
if board[i][col] == num:
return False
# 定义小方格的左上角坐标
box_x = (row // 3) * 3
box_y = (col // 3) * 3
# 检查小方格内是否已有相同的数字
for i in range(box_x, box_x + 3):
for j in range(box_y, box_y + 3):
if board[i][j] == num:
return False
return True
def solve_sudoku(board):
for i in range(9):
for j in range(9):
if board[i][j] == 0: # 如果当前单元格为空
for num in range(1, 10): # 遍历1到9的所有数字
if is_valid(board, i, j, num):
board[i][j] = num # 尝试填入数字
if solve_sudoku(board): # 继续递归地寻找解决方案
return True
else: # 如果无法找到解决方案,回溯并尝试下一个数字
board[i][j] = 0
return False # 当所有数字都尝试过,无法找到解,返回False
return True # 找到了完整的解,返回True
# 初始化一个9x9的空矩阵作为数独游戏板
board = [
[5, 3, 0, 0, 7, 0, 0, 0, 0],
[6, 0, 0, 1, 9, 5, 0, 0, 0],
[0, 9, 8, 0, 0, 0, 0, 6, 0],
[8, 0, 0, 0, 6, 0, 0, 0, 3],
[4, 0, 0, 8, 0, 3, 0, 0, 1],
[7, 0, 0, 0, 2, 0, 0, 0, 6],
[0, 6, 0, 0, 0, 0, 2, 8, 0],
[0, 0, 0, 4, 1, 9, 0, 0, 5],
[0, 0, 0, 0, 8, 0, 0, 7, 9]
]
if solve_sudoku(board):
print("Sudoku solved:")
for row in board:
print(row)
else:
print("No solution exists.")
python编程实现一个八数码问题的棋盘可视化,以及移动棋盘的判断和棋盘的判断功能。
好的,下面是实现八数码问题的棋盘可视化,移动棋盘的判断和棋盘的判断的Python代码:
```python
class EightPuzzle:
def __init__(self, board):
self.board = board
def move(self, direction):
empty_slot = self.board.index(0)
if direction == 'up':
if empty_slot not in [0, 1, 2]:
self.board[empty_slot], self.board[empty_slot - 3] = self.board[empty_slot - 3], self.board[empty_slot]
return True
elif direction == 'down':
if empty_slot not in [6, 7, 8]:
self.board[empty_slot], self.board[empty_slot + 3] = self.board[empty_slot + 3], self.board[empty_slot]
return True
elif direction == 'left':
if empty_slot not in [0, 3, 6]:
self.board[empty_slot], self.board[empty_slot - 1] = self.board[empty_slot - 1], self.board[empty_slot]
return True
elif direction == 'right':
if empty_slot not in [2, 5, 8]:
self.board[empty_slot], self.board[empty_slot + 1] = self.board[empty_slot + 1], self.board[empty_slot]
return True
return False
def is_solved(self):
return self.board == [0, 1, 2, 3, 4, 5, 6, 7, 8]
def visualize(self):
for i in range(0, 9, 3):
print(self.board[i:i + 3])
```
这个类实现了以下方法:
- `__init__`: 初始化八数码棋盘;
- `move`: 移动棋子,判断是否能够移动;
- `is_solved`: 判断当前棋盘是否为解决方案;
- `visualize`: 可视化棋盘。
下面是一个使用示例:
```python
board = [1, 2, 3, 4, 0, 5, 6, 7, 8]
puzzle = EightPuzzle(board)
puzzle.visualize() # 输出 [1, 2, 3] [4, 0, 5] [6, 7, 8]
puzzle.move('up')
puzzle.visualize() # 输出 [1, 0, 3] [4, 2, 5] [6, 7, 8]
puzzle.move('left')
puzzle.visualize() # 输出 [0, 1, 3] [4, 2, 5] [6, 7, 8]
print(puzzle.is_solved()) # 输出 False
```
在这个例子中,我们创建了一个初始棋盘,可视化并且移动了两个棋子。然后我们检查了当前棋盘是否为解决方案。
阅读全文
相关推荐
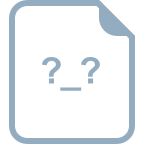
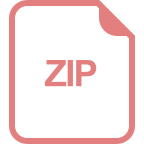
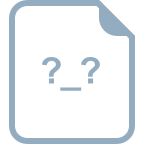
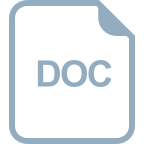
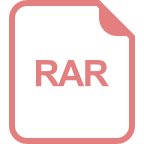
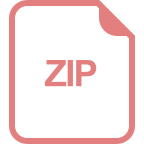
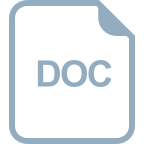
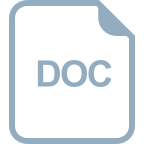
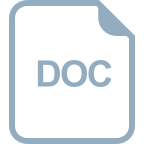
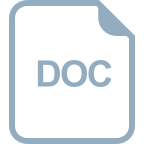
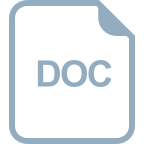
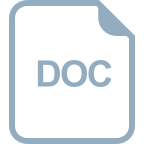
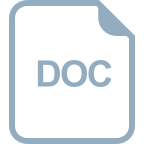
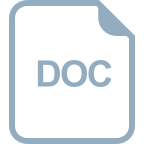
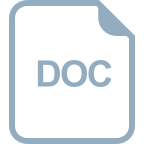
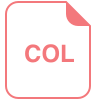