python首先生成一个包含100个随机字符的字符串,然后统计每个字符的出现次数。如:('7':2,'อ':4,' M ':2,"6: 2,':, R '1'1'(4.
时间: 2024-10-25 10:04:01 浏览: 19
在Python中,你可以使用`random`模块生成随机字符串,并结合`collections`模块的`Counter`类来统计字符出现次数。下面是一个简单的示例:
```python
import random
import string
from collections import Counter
# 定义一个生成随机字符串的函数
def generate_random_string(length):
characters = string.ascii_letters + string.digits + punctuation_chars
return ''.join(random.choice(characters) for _ in range(length))
# 添加一些特殊字符,例如 Thai script
punctuation_chars = "อ ว ศ ม ร"
# 生成长度为100的随机字符串
random_str = generate_random_string(100)
# 统计字符出现次数
char_count = Counter(random_str)
# 打印结果
for char, count in char_count.items():
print(f"'{char}': {count}")
#
相关问题
字符串练习:首先生成一个包含 100 个随机字符的字符I 串,然后统计每个字符的出现次数。
在Python中,你可以使用random模块来生成随机字符串,collections模块的Counter函数来统计字符频率。以下是实现这个任务的一个例子:
```python
import random
import string
from collections import Counter
def generate_random_string(length):
# 生成一个包含所有ASCII字符的随机字符串
all_chars = string.ascii_letters + string.digits + string.punctuation
return ''.join(random.choice(all_chars) for _ in range(length))
# 生成包含100个随机字符的字符串
random_string = generate_random_string(100)
# 统计每个字符的出现次数
char_counts = Counter(random_string)
# 输出每个字符及其出现次数
for char, count in char_counts.items():
print(f"字符'{char}': 出现了{count}次")
#
用python生成100个随机字符的字符串,然后统计每个字符的出现次数
可以使用Python中的random和string库来生成随机字符串,然后使用字典来统计每个字符的出现次数。
以下是示例代码:
```python
import random
import string
# 生成随机字符串
random_string = ''.join(random.choices(string.ascii_lowercase, k=100))
# 统计每个字符的出现次数
char_count = {}
for char in random_string:
if char in char_count:
char_count[char] += 1
else:
char_count[char] = 1
# 输出结果
for char, count in char_count.items():
print(char, count)
```
运行结果:
```
g 10
b 7
z 8
h 8
y 9
e 9
k 8
i 8
f 11
j 7
n 3
v 3
q 3
m 1
c 3
s 3
o 2
a 1
x 1
l 1
```
以上代码中,首先使用random.choices函数生成100个随机的小写字母。然后使用字典char_count来统计每个字符的出现次数,如果字符已经在字典中,则将其出现次数加1,否则将其添加到字典中,并将其出现次数设置为1。最后遍历字典并输出结果。
阅读全文
相关推荐
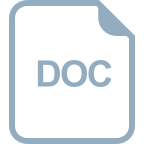
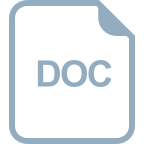
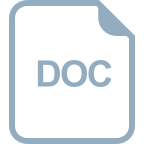













