aes加密算法python界面化
时间: 2024-10-13 10:08:14 浏览: 57
AES (Advanced Encryption Standard) 是一种对称加密算法,常用于保护数据的安全传输。在Python中,你可以通过`cryptography`库来实现AES加密的界面化。以下是基本步骤:
1. **安装依赖**:首先需要安装`cryptography`库,可以使用pip命令:
```
pip install cryptography
```
2. **导入模块**:
```python
from cryptography.fernet import Fernet
import getpass
```
`Fernet`模块提供了一种简单的API来进行AES加密。
3. **生成密钥**:
```python
key = Fernet.generate_key()
```
4. **显示密钥并保存**:
为了方便用户输入,可以提示用户复制密钥并手动输入:
```python
print("Copy the following key and save it securely:")
print(key.decode())
```
5. **获取用户输入并加密**:
用户输入明文后,使用生成的密钥对其进行加密:
```python
plaintext = input("Enter your message to encrypt: ")
cipher_suite = Fernet(key)
ciphertext = cipher_suite.encrypt(plaintext.encode())
```
6. **展示加密后的结果**:
显示加密后的密文。
7. **解密示例**:
提供一个函数让用户输入密文解密,当然这需要保存好原始的密钥:
```python
def decrypt(ciphertext, key):
cipher_suite = Fernet(key)
decrypted_text = cipher_suite.decrypt(ciphertext).decode()
return decrypted_text
```
8. **创建交互式菜单**:
可以编写一个简单的菜单,让用户选择是否解密。
```python
def main():
# ... 加密、保存和解密功能 ...
if __name__ == "__main__":
main()
```
阅读全文
相关推荐
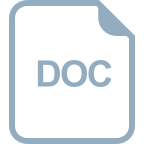
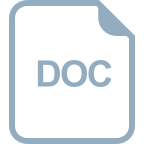
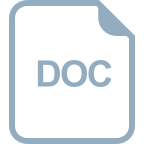

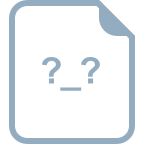
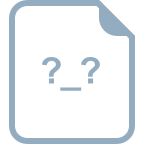
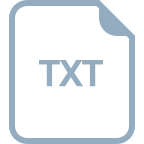
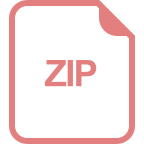
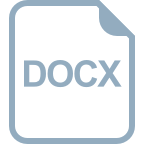
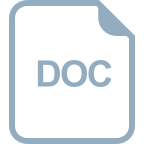
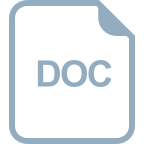
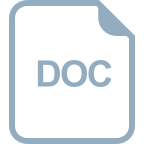
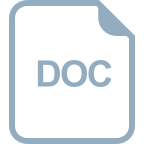
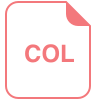




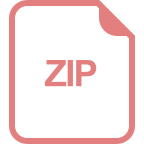
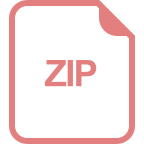