element_to_be_clickable 用法
时间: 2024-10-05 17:02:41 浏览: 125
`element_to_be_clickable()` 是Selenium WebDriver中一个用于等待元素变为可点击的状态的断言方法。这个方法通常在JavaScript中作为`ExpectedConditions`的一部分使用,它会在给定的元素存在并且可见的前提下,进一步检查该元素是否处于可以响应用户点击的行为状态。
例如,如果你有一个元素,你想确保它加载完成后并且在可见范围内,然后再尝试点击,你可以这样做:
```python
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.common.by import By
from selenium.webdriver.support import expected_conditions as EC
element = driver.find_element(By.ID, "your-element-id")
wait = WebDriverWait(driver, 10) # 设置超时时间
wait.until(EC.element_to_be_clickable(element)) # 等待元素变得可点击
# 现在你可以安全地调用click()方法
element.click()
```
如果元素在指定时间内未变成可点击,`wait.until()`会抛出异常。
相关问题
element_to_be_clickable 使用示例
下面是使用`element_to_be_clickable`等待条件的一个示例:
```python
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
# 创建浏览器对象
driver = webdriver.Chrome()
# 打开网页
driver.get("https://www.example.com")
# 设置等待时间
wait = WebDriverWait(driver, 10)
# 等待元素可点击
element = wait.until(EC.element_to_be_clickable((By.ID, "button_id")))
# 执行点击操作
element.click()
# 关闭浏览器
driver.quit()
```
在上述示例中,首先创建一个浏览器对象,然后打开网页。接下来,使用`WebDriverWait`设置等待时间为10秒,并使用`element_to_be_clickable`等待条件来等待具有指定ID的按钮元素可被点击。一旦元素变为可点击状态,就会执行`click()`方法进行点击操作。最后,关闭浏览器。
请注意,示例中的`button_id`需要替换为实际页面中按钮元素的ID。此外,还可以使用其他定位方式(如`By.XPATH`、`By.CSS_SELECTOR`等)来指定元素的定位方式。
使用element_to_be_clickable修改代码
如果你想在2秒后不管元素是否可点击都继续执行后续操作,你可以直接使用`element_to_be_clickable`方法,它本身包含了超时机制,不会增加额外的隐式等待。下面是修改后的代码片段:
```python
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
start_time = time.time()
play_button = WebDriverWait(driver, 2).until(EC.element_to_be_clickable((By.XPATH, play_button_XPATH)))
if play_button:
print("存在播放按钮")
else:
end_time = time.time()
print("\n",interval_to_str(end_time - start_time))
print("不存在播放按钮")
# 如果这里不需要异常处理,可以去掉 try-except 块
```
这样,如果在2秒内找不到可点击的元素,程序会直接跳过找到按钮的部分,并打印出"不存在播放按钮"以及等待的具体时间。如果找到可点击的元素,则按预期执行后续逻辑。由于没有了`TimeoutException`处理,因此代码的流程会有所不同。
阅读全文
相关推荐
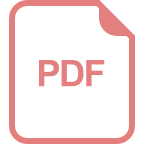
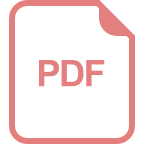
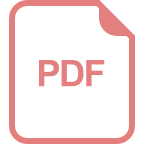













