2个乒乓球队进行比赛,各出3人,甲队为A、B、C3人;乙队为X、Y、Z3人。 抽签决定比赛名单。有人向队员打听比赛的名单,A说他不和X比,C说他不和X、Z比 编程决定比赛名单。
时间: 2024-11-29 21:35:05 浏览: 13
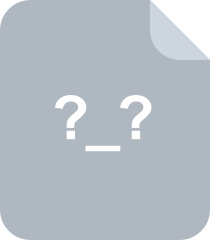
python 两个乒乓球队进行比赛,各出三人,找出三队赛手的名单(示例)

要编程确定比赛名单,我们可以使用一个简单的逻辑来匹配球员之间的配对,同时确保满足A和C的要求。我们可以创建一个二维数组或矩阵来存储可能的比赛组合,然后根据条件排除不适合的组合。以下是用C语言编写的简单示例:
```c
#include <stdio.h>
#define NUM_PLAYERS 3
#define NUM_MATCHES 3
// 假设match[match_index][player_index]表示第match_index场比赛的第player_index名选手
int match[NUM_MATCHES][NUM_PLAYERS];
void generateMatches(char team1[][3], char team2[][3]) {
for (int i = 0; i < NUM_MATCHES; i++) {
int availablePlayers1 = 0, availablePlayers2 = 0;
// 遍历团队成员,检查哪些没有被指定不能对战的人
for (int j = 0; j < NUM_PLAYERS; j++) {
if (strcmp(team1[i], "A") != 0 && strcmp(team2[i], "X") != 0) availablePlayers1++;
if (strcmp(team1[i], "C") != 0 && strcmp(team2[i], "X") != 0 && strcmp(team1[i], "Z") != 0) availablePlayers2++;
}
if (availablePlayers1 == 0 || availablePlayers2 == 0) {
printf("Error: No valid match found for player %s vs %s\n", team1[i], team2[i]);
continue;
}
// 如果双方都有可用选手,随机选择一个
for (int j = 0; j < NUM_PLAYERS; j++) {
if (team1[i][j] != 'X' && team2[i][j] != 'X') {
match[i][0] = team1[i][j];
match[i][1] = team2[i][j];
break;
}
}
// 对于剩余的两个位置,从剩下的选手中随机选择
for (int j = 0; j < NUM_PLAYERS - 1; j++) {
if (team1[i][j] == match[i][0] || team1[i][j] == match[i][1])
continue;
match[i][j + 1] = team1[i][j];
}
// 检查是否还有剩余选手,如果有,则选择乙队
if (j < NUM_PLAYERS - 1) {
for (int j = 0; j < NUM_PLAYERS; j++) {
if (team2[i][j] != match[i][0] && team2[i][j] != match[i][1]) {
match[i][j + 1] = team2[i][j];
break;
}
}
}
}
}
int main() {
char team1[3][3] = {"A", "B", "C"};
char team2[3][3] = {"X", "Y", "Z"};
generateMatches(team1, team2);
// 输出比赛名单
for (int i = 0; i < NUM_MATCHES; i++) {
printf("Match %d: %s vs %s\n", i+1, match[i][0], match[i][1]);
}
return 0;
}
```
这个程序首先初始化两个队伍的成员,然后调用`generateMatches`函数生成比赛名单。注意,这里假设没有更多的限制条件(例如A不能和B对决等),只是基于A和C的声明进行了安排。如果存在其他条件,可以在`generateMatches`函数中添加相应的判断。
阅读全文
相关推荐
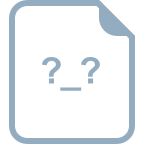
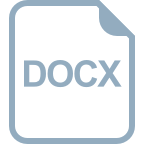















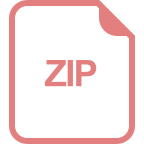