1、初始化单链表; 2、单链表的插入; 3、单链表的输出; 4、求单链表的长度; 5、判断单链表是否为空; 6、输出单链表的第i位置的元素; 7、在单链表中查找一个给定元素在表中的位置; 8、单链表的删除; 9、释放单链表
时间: 2024-10-12 11:03:41 浏览: 33
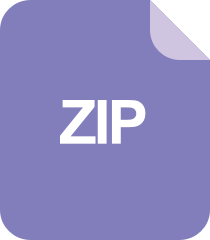
使用C++实现单链表的基本操作:1、创建单链表2、遍历单链表3、单链表插入4、删除单链表5、判断是否为空6、单链表的

1. **初始化单链表**:
如果是空链表:
```c
typedef struct Node{
int data;
struct Node* next;
}Node;
Node* head = NULL;
```
如果需要初始一个带有一个或多个节点的链表,可以动态分配内存创建首节点:
```c
Node* head = (Node*) malloc(sizeof(Node));
head->data = some_value;
head->next = NULL;
```
2. **单链表插入**:
- 在头部插入: `newNode->next = head; head = newNode;`
- 在中间或尾部插入: 需要先找到插入位置,然后修改相应节点的`next`指针。
3. **单链表输出**:
```c
void displayList(Node* head){
if(head == NULL)
printf("链表为空");
else{
while(head != NULL){
printf("%d ->", head->data);
head = head->next;
}
printf("NULL");
}
}
```
4. **求单链表长度**:
通常需要辅助变量:
```c
int length(Node* head){
int count = 0;
while(head != NULL){
count++;
head = head->next;
}
return count;
}
```
5. **判断单链表是否为空**:
```c
bool isEmpty(Node* head){
return head == NULL;
}
```
6. **输出单链表的第i位置的元素**:
需要实现循环或递归遍历找到对应位置:
```c
void getNthElement(Node* head, int i, int& result){
int index = 0;
while(head && index < i){
head = head->next;
index++;
}
if(index == i)
result = head->data;
else
result = -1; // 表示越界
}
```
7. **查找元素位置**:
```c
int searchElement(Node* head, int target){
int position = 0;
while(head != NULL){
if(head->data == target)
return position;
head = head->next;
position++;
}
return -1; // 元素未找到
}
```
8. **单链表删除**:
删除某个节点通常涉及两个步骤:找到要删除的节点以及处理其后的链接:
```c
void deleteNode(Node** head, int key){
Node* temp = *head, *prev = NULL;
while(temp != NULL && temp->data != key){
prev = temp;
temp = temp->next;
}
if(temp == NULL) return; // 要删除的节点不存在
if(prev == NULL) *head = temp->next; // 删除头节点
else prev->next = temp->next;
free(temp); // 释放内存
}
```
9. **释放单链表**:
清理所有节点:
```c
void destroyList(Node** head){
Node* current = *head;
while(current != NULL){
Node* nextTemp = current->next;
free(current);
current = nextTemp;
}
*head = NULL;
}
```
阅读全文
相关推荐
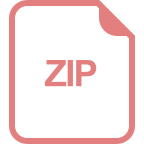
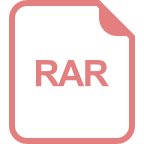








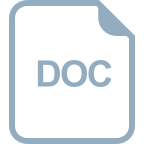






