编写完整的C++语言程序完成下列实验任务:定义顺序存储的串类,完成下列各功能(要求不能使用strcmp、strcpy等等库函数来直接实现): 1、求子串。返回从主串s的第i个字符开始长为len的子串。 主串s的值、i、len等值均自定。要求有结果输出。(下同)
时间: 2024-10-25 21:03:31 浏览: 9
在C++中,我们可以定义一个简单的字符串类`MyString`来完成这个任务。由于不允许使用标准库中的字符串处理函数,我们将手动创建一个`substring`方法来提取子串。以下是完整的C++代码:
```cpp
#include <iostream>
class MyString {
private:
char* str;
int length;
public:
// 构造函数
MyString(const char* s) : str(new char[strlen(s) + 1]), length(strlen(s)) {
std::copy(s, s + length, str);
str[length] = '\0'; // 添加终止符
}
~MyString() { delete[] str; } // 析构函数释放内存
// 求子串
MyString substring(int i, int len) const {
if (i < 0 || i >= length || len <= 0 || i + len > length) {
return MyString(""); // 如果索引超出范围,返回空串
}
int newLength = len;
char* subStr = new char[newLength + 1];
std::copy(str + i, str + i + len, subStr); // 复制子串到新内存
subStr[newLength] = '\0';
MyString result(subStr);
delete[] subStr;
return result;
}
// 输出字符串
void print() const {
for (int i = 0; i < length; ++i) {
std::cout << str[i];
}
std::cout << std::endl;
}
};
int main() {
char mainStr[] = "Hello, C++!";
int i = 7, len = 5; // 要获取的子串起始位置和长度
MyString s(mainStr);
std::cout << "Main string: ";
s.print();
MyString subStr = s.substring(i, len);
std::cout << "Substring from position " << i << " to " << i+len-1 << ": ";
subStr.print();
return 0;
}
```
当你运行此程序时,它会先打印出原始字符串"Hello, C++!",然后输出指定位置和长度的子串。
阅读全文
相关推荐
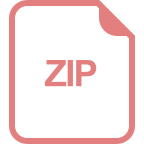
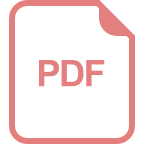
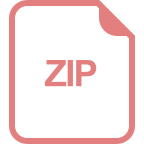

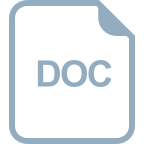
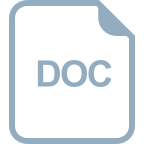
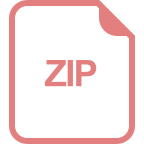
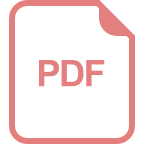
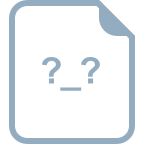
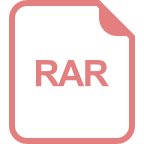
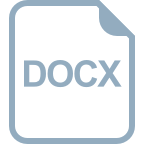
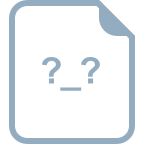
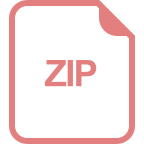
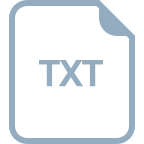
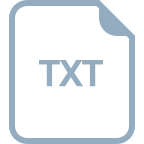
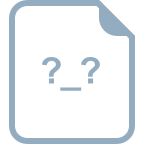
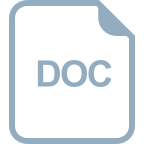
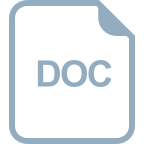