编写单链表的删除操作函数,完整代码
时间: 2024-09-11 15:10:50 浏览: 42
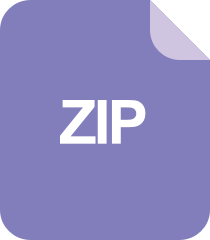
cpp代码-单链表的操作-oj
单链表的删除操作函数通常需要处理三种情况:删除头节点、删除中间节点、以及删除尾节点。下面是一个简单的单链表节点定义以及删除节点的函数实现,使用的是C语言:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义单链表的节点结构体
typedef struct ListNode {
int val;
struct ListNode *next;
} ListNode;
// 创建一个新的链表节点
ListNode* createNode(int value) {
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
if (!newNode) {
return NULL;
}
newNode->val = value;
newNode->next = NULL;
return newNode;
}
// 删除链表中值为value的节点
void deleteNode(ListNode** head, int value) {
if (head == NULL || *head == NULL) {
return;
}
ListNode* current = *head;
ListNode* previous = NULL;
// 如果头节点就是要删除的节点
if (current != NULL && current->val == value) {
*head = current->next;
free(current);
return;
}
// 查找要删除的节点
while (current != NULL && current->val != value) {
previous = current;
current = current->next;
}
// 如果没有找到,返回
if (current == NULL) {
return;
}
// 删除节点
previous->next = current->next;
free(current);
}
// 打印链表
void printList(ListNode* head) {
ListNode* current = head;
while (current != NULL) {
printf("%d ", current->val);
current = current->next;
}
printf("\n");
}
// 释放链表内存
void freeList(ListNode* head) {
ListNode* current = head;
while (current != NULL) {
ListNode* next = current->next;
free(current);
current = next;
}
}
int main() {
// 创建链表 1 -> 2 -> 3 -> 4
ListNode* head = createNode(1);
head->next = createNode(2);
head->next->next = createNode(3);
head->next->next->next = createNode(4);
printf("原始链表: ");
printList(head);
// 删除节点
deleteNode(&head, 3); // 删除值为3的节点
printf("删除节点后: ");
printList(head);
// 释放链表内存
freeList(head);
return 0;
}
```
这段代码中定义了一个简单的单链表节点结构体`ListNode`,并提供了创建节点、删除节点、打印链表和释放链表内存的函数。`deleteNode`函数接受链表头节点的地址和要删除节点的值,根据值来删除对应的节点。
阅读全文
相关推荐
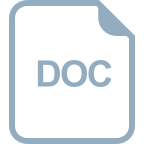
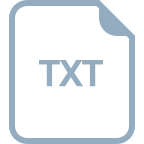


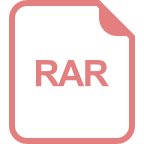
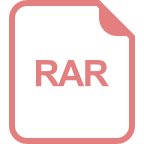
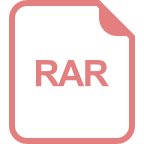
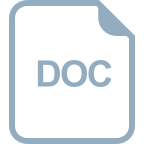









