c#实现PLC与上位机交互代码
时间: 2024-09-20 17:09:31 浏览: 64
在C#中,通过.NET Framework中的一些库,比如System.IO.Ports或PLCNet等,可以实现PLC(可编程逻辑控制器)与上位机(通常指计算机)的数据交换。以下是一个简单的例子,展示了如何使用System.IO.Ports库连接到串口PLC并发送接收数据:
```csharp
using System;
using System.IO.Ports;
class PLCCommunication
{
private SerialPort plcSerial;
public PLCCommunication(string portName, int baudRate)
{
plcSerial = new SerialPort(portName, baudRate);
plcSerial.Open();
}
// 发送数据函数
public void SendData(string data)
{
try
{
plcSerial.Write(data);
Console.WriteLine("Sent: " + data);
}
catch (Exception ex)
{
Console.WriteLine("Error sending data: " + ex.Message);
}
}
// 接收数据函数
public string GetData()
{
byte[] buffer = new byte[10]; // 假设接收最多10字节
int receivedBytes = plcSerial.Read(buffer, 0, buffer.Length);
if (receivedBytes > 0)
{
return Encoding.ASCII.GetString(buffer, 0, receivedBytes);
}
else
{
return "No data received";
}
}
// 关闭连接
public void Close()
{
plcSerial.Close();
}
}
public class Program
{
static void Main(string[] args)
{
PLCCommunication plc = new PLCCommunication("COM1", 9600); // 连接示例,替换为实际的端口号和波特率
try
{
plc.SendData("Command"); // 发送命令
string response = plc.GetData(); // 接收响应
Console.WriteLine("Received: " + response);
}
finally
{
plc.Close(); // 确保关闭连接
}
}
}
```
阅读全文
相关推荐
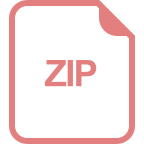
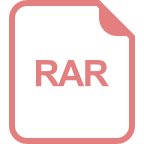
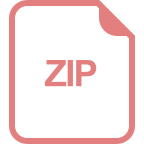

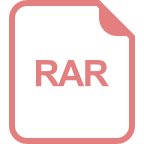
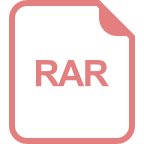
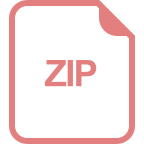
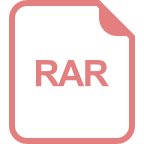
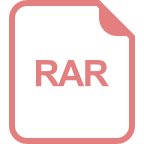
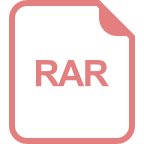
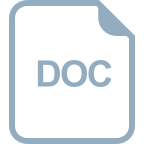
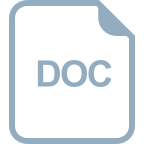
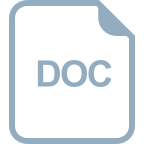
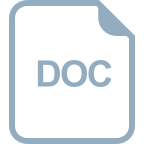
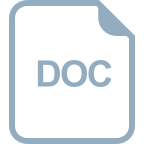
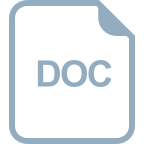
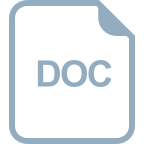
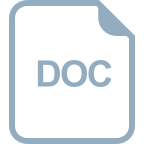
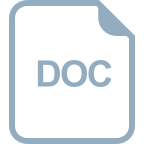