python img 切片
时间: 2023-10-13 21:26:14 浏览: 100
在 Python 中,你可以使用 PIL(Python Imaging Library) 或者 OpenCV 来对图像进行切片。下面是使用 PIL 进行图像切片的示例代码:
```python
from PIL import Image
# 打开图像
image = Image.open('image.jpg')
# 定义切片范围
left = 100
top = 100
right = 300
bottom = 300
# 切片图像
cropped_image = image.crop((left, top, right, bottom))
# 显示切片后的图像
cropped_image.show()
```
在上述示例中,我们首先使用 `Image.open()` 方法打开了一张图像。然后定义了切片的区域范围,即左上角的坐标 `(left, top)` 和右下角的坐标 `(right, bottom)`。接着使用 `crop()` 方法对图像进行切片,并将结果保存在 `cropped_image` 变量中。最后,使用 `show()` 方法显示切片后的图像。
请注意,你需要将上述示例中的 `'image.jpg'` 替换为你要切片的实际图像的路径。
相关问题
python图像切片
Python图像切片是将一张大图片切割成多个小图片的过程。在Python中,可以使用PIL库来实现图像切片。下面是两种常用的图像切片方法:
方法一:通过四个坐标点在任意位置切割图片
```python
from PIL import Image
def cut_image(path):
img = Image.open(path)
w, h = img.size
cut = [(0, 0, 120, h), (120, 0, 240, h), (240, 0, 360, h), (360, 0, w, h)]
for i, n in enumerate(cut, 1):
temp = img.crop(n)
temp.save(path.replace(".jpg", str(i - 1) + '.jpg'))
return True
```
方法二:通过坐标的最大最小值对图片进行整体切割
```python
from PIL import Image
def cut_img_by_xy(path1, x_min, x_max, y_min, y_max, path2):
img = Image.open(path1)
crop = img.crop((x_min, y_min, x_max, y_max))
crop.save(path2)
```
这两种方法可以根据需求调整切割的位置和大小。你可以根据需要选择其中一种方法来进行图像切片。
请问还有其他问题吗?
Python中用切片的方式如何获取到一张图片斜对角线的像素
在Python中,特别是使用PIL(Pillow)库处理图像时,如果你想通过切片获取一张图片的斜对角线像素,你需要先将图像转换为一维数组,这通常是通过将图像矩阵沿着指定方向(例如从左上角到右下角)进行扁平化来实现。然后你可以使用切片操作来提取特定的斜对角线部分。
以下是一个简单的示例:
```python
from PIL import Image
# 打开并加载图片
img = Image.open('your_image.jpg')
# 将图片转换为灰度图像,如果原图不是灰度,可以根据需要进行选择
gray_img = img.convert('L')
# 获取图片尺寸
width, height = gray_img.size
# 计算斜对角线的最大长度(取宽度和高度的较小值)
max_diagonal_len = min(width, height)
# 创建一个空列表用于存储像素值
diagonal_pixels = []
# 使用切片获取斜对角线像素
for y in range(max_diagonal_len):
x = y
diagonal_pixels.append(gray_img.getpixel((x, y)))
# 如果需要,可以将其转换回原始形状或进一步处理这些像素
```
注意,这个例子假设是从左上角到右下角的斜对角线。如果你想要其他方向的斜对角线,只需调整`x`和`y`的关系即可。
阅读全文
相关推荐
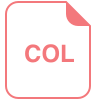
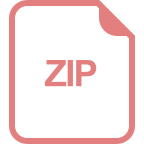
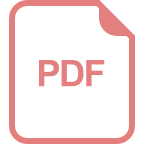
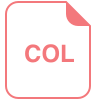
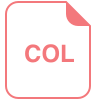
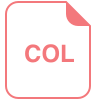







