def convert(size, box): if size[0] != 0: dw = 1. / size[0] else: dw = 0 # 或者你可以选择其他的处理方式 if size[0] != 0: dh = 1. / size[1] else: dw = 0 x = (box[0] + box[1]) / 2.0 y = (box[2] + box[3]) / 2.0 w = box[1] - box[0] h = box[3] - box[2] x = x * dw w = w * dw y = y * dh h = h * dh return (x, y, w, h) 请你根据报错修改这段代码 Traceback (most recent call last): File "D:\hyh\pythonProject\main.py", line 148, in <module> convert_annotation(nameWithoutExtention) # convert label File "D:\hyh\pythonProject\main.py", line 66, in convert_annotation bb = convert((w, h), b) File "D:\hyh\pythonProject\main.py", line 43, in convert y = y * dh UnboundLocalError: local variable 'dh' referenced before assignment 这是报错
时间: 2024-04-17 18:24:17 浏览: 202
根据报错信息,这段代码中的错误是 `UnboundLocalError: local variable 'dh' referenced before assignment`,即在使用变量 `dh` 之前没有对其进行赋值。根据代码逻辑,我发现在第 6 行和第 10 行的赋值语句中,错误地将 `dw` 赋值给了 `dh`。下面是修改后的代码:
```python
def convert(size, box):
if size[0] != 0:
dw = 1. / size[0]
else:
dw = 0
if size[1] != 0: # 修改此处判断条件
dh = 1. / size[1]
else:
dh = 0
x = (box[0] + box[1]) / 2.0
y = (box[2] + box[3]) / 2.0
w = box[1] - box[0]
h = box[3] - box[2]
x = x * dw
w = w * dw
y = y * dh
h = h * dh
return (x, y, w, h)
```
这样修改后,再次运行代码应该就不会出现该报错了。
相关问题
def convert(size, box): if size[0] != 0: dw = 1. / size[0] else: dw = 0 # 或者你可以选择其他的处理方式 if size[0] != 0: dh = 1. / size[1] else: dw = 0 x = (box[0] + box[1]) / 2.0 y = (box[2] + box[3]) / 2.0 w = box[1] - box[0] h = box[3] - box[2] x = x * dw w = w * dw y = y * dh h = h * dh return (x, y, w, h) 请你根据报错修改这段代码
根据代码中的报错信息,可以看出第8行和第12行的变量名写错了。应该将`dw`改为`dh`,将``改为`dw`。修改后的代码如下:
```python
def convert(size, box):
if size[0] != 0:
dw = 1. / size[0]
else:
dw = 0
# 或者你可以选择其他的处理方式
if size[1] != 0:
dh = 1. / size[1]
else:
dh = 0
x = (box[0] + box[1]) / 2.0
y = (box[2] + box[3]) / 2.0
w = box[1] - box[0]
h = box[3] - box[2]
x = x * dw
w = w * dw
y = y * dh
h = h * dh
return (x, y, w, h)
```
这样修改后的代码就纠正了变量名错误,并且可以正常运行了。
def convert(size, box): dw = 1. / size[0] dh = 1. / size[1] x = (box[0] + box[1]) / 2.0 y = (box[2] + box[3]) / 2.0 w = box[1] - box[0] h = box[3] - box[2] x = x * dw w = w * dw y = y * dh h = h * dh return (x, y, w, h) 怎么修改呢? Traceback (most recent call last): File "D:\hyh\pythonProject\main.py", line 134, in <module> convert_annotation(nameWithoutExtention) # convert label File "D:\hyh\pythonProject\main.py", line 58, in convert_annotation bb = convert((w, h), b) File "D:\hyh\pythonProject\main.py", line 27, in convert dw = 1. / size[0] ZeroDivisionError: float division by zero 这是完整报错
根据报错信息来看,这里出现了除数为0的错误。体是在 `convert` 函数中的 `dw = 1. / size[0]` 这一行代码导致的。
为了解决这个问题,你可以在进行除法操作之前,先判断一下除数是否为0。可以使用一个条件语句来进行判断,如下所示:
```python
def convert(size, box):
if size[0] != 0:
dw = 1. / size[0]
else:
dw = 0
# 或者你可以选择其他的处理方式
dh = 1. / size[1]
x = (box[0] + box[1]) / 2.0
y = (box[2] + box[3]) / 2.0
w = box[1] - box[0]
h = box[3] - box[2]
x = x * dw
w = w * dw
y = y * dh
h = h * dh
return (x, y, w, h)
```
通过这样的修改,当 `size[0]` 等于0时,可以避免出现除以零的错误。
阅读全文
相关推荐
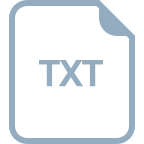
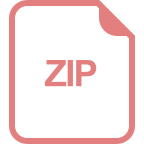
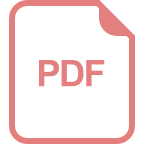
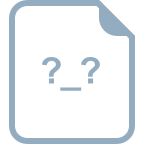
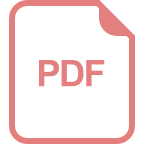
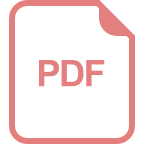
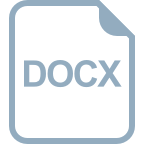
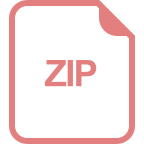
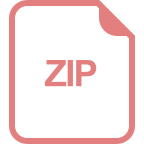
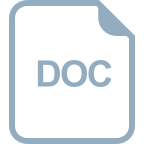
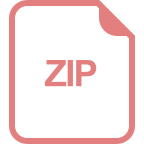
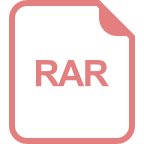
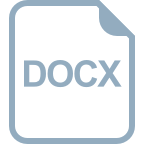
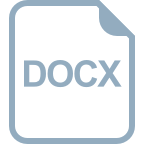
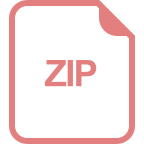
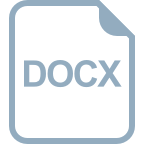